The data should be converted to json or a javascript object like this:
var xlsData = {
"RedRect": "This is the Red Rectangle!",
"Star": "This is the Star Shape!"
}
The best way is to use the javascript event load on a svg object to attach the mouse events. Because jQuery prevents to bind load events to object elements, we have to use javascript addEventListener
to setup the load event.
How to listen to a load event of an object with a SVG image?
Inside the SVG file, we have two objects with the ids RedRect
and Star
:
<rect id="RedRect" x="118" y="131" class="st0" width="153" height="116"/>
<polygon id="Star" class="st2" points="397,252.3 366.9,245.4 344.2,266.3 341.5,235.6 314.6,220.4 343,208.3 349.1,178.1
369.4,201.4 400,197.8 384.2,224.3 "/>
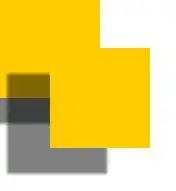
Now, all we have to do is attach our events when the svg objects loads:
<object id="svg" type="image/svg+xml" data="test-links.svg">Your browser does not support SVG</object>
$('object')[0].addEventListener('load', function() {
$('#RedRect', this.contentDocument).on({
'mouseenter': function() {
$('#hover-status').text('#svg #RedRect Mouse Enter');
$('#hover-data').text(xlsData['RedRect']);
},
'mouseleave': function() {
$('#hover-status').text('#svg #RedRect Mouse Leave');
$('#hover-data').html(' ');
}
});
$('#Star', this.contentDocument).on({
'mouseenter': function() {
$('#hover-status').text('#svg #Star Mouse Enter');
$('#hover-data').text(xlsData['Star']);
},
'mouseleave': function() {
$('#hover-status').text('#svg #Star Mouse Leave');
$('#hover-data').html(' ');
}
});
}, true);
Plunker example