The FuncFormatter gives you a very flexible way to define your own (e.g. dynamic) tick label formatting to an axis.
Your custom function should accept x and pos parameters, where pos is the (positional) number of the tick label that is currently being formatted, and the x is the actual value to be (pretty) printed.
In that respect, the function gets called each time a visible tick mark should be generated - that's why you always get a sequence of function calls with positional argument starting from 1 to the maximal number of visible arguments for your axis (with their corresponding values).
Try running this example, and zoom the plot:
from matplotlib.ticker import FuncFormatter
import matplotlib.pyplot as plt
import numpy as np
x = np.arange(4)
y = x**2
def MyTicks(x, pos):
'The two args are the value and tick position'
if pos is not None:
tick_locs=ax.yaxis.get_majorticklocs() # Get the list of all tick locations
str_tl=str(tick_locs).split()[1:-1] # convert the numbers to list of strings
p=max(len(i)-i.find('.')-1 for i in str_tl) # calculate the maximum number of non zero digit after "."
p=max(1,p) # make sure that at least one zero after the "." is displayed
return "pos:{0}/x:{1:1.{2}f}".format(pos,x,p)
formatter = FuncFormatter(MyTicks)
fig, ax = plt.subplots()
ax.yaxis.set_major_formatter(formatter)
plt.plot(x,y,'--o')
plt.show()
The result should look like this:
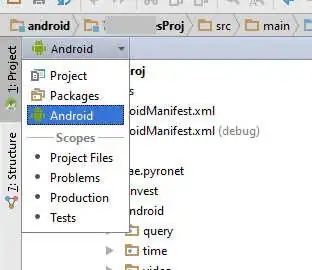