Okay, there are many approaches for this. I will explain one such approach.
Step 1: Create StudentReport class.
Notice the byte[] array used here.
public class StudentReport {
private long id;
private String Name;
byte[] report;
public long getId() {
return id;
}
public void setId(long id) {
this.id = id;
}
public String getName() {
return Name;
}
public void setName(String name) {
Name = name;
}
public byte[] getReport() {
return report;
}
public void setReport(byte[] report) {
this.report = report;
}
}
Step 2 : Create the required rest end point.
Here I wish to tell you few things.
The Json has to be send to end point as string and keep the report empty there. This will be passed seperatley like shown below.
@RestController
@RequestMapping("/NameYourController/")
public class MyController{
@RequestMapping(value = "addDetails", method = RequestMethod.POST , consumes = "multipart/form-data")
public StudentReport addProduct(@RequestParam String studentReportJson, @RequestParam MultipartFile report) throws JsonParseException, JsonMappingException, IOException {
//Convert your Json in Strign format as actual object
StudentReport studentReport = new ObjectMapper().readValue(studentReportJson, StudentReport.class);
//convert file to byte array
byte[] myReport = report.getBytes();
studentReport.setReport(myReport);
// Now, pdf is set in the object
// do whatever you want to do with it like save in database etc
//based on that , have some return type defined. I have just used returned the object in this example .
return studentReport; // Spring boot auto converts this object to Json when send to UI.Note that Json is an object and Json String is string object consisting of Json. We recieved Json String but returning Json object
}
//and other endpoints you want to define
}
That's it done. Note that for this you need to import the Jackson library. If you are using Maven, you can add this in your pom.xml.
<dependency>
<groupId>org.codehaus.jackson</groupId>
<artifactId>jackson-mapper-asl</artifactId>
<version>1.9.12</version>
</dependency>
Now, how would you test your rest end point. One way will be from the UI code which will consume these.But then be sure of that code that it is correct. If you don't have UI code still you can do this. This is done by chrome app PostMan. But, for that you need to use chrome web browser.
Youtube have lots of videos on how to use PostMan. I will just give you a screen shot of how to use specifically in your case :
Notice these things in image -> POST, URL Path, BODY , form-data, TEXT , FILE. :)
Output will be displayed below in postman if you have some return data.(not shown in image)
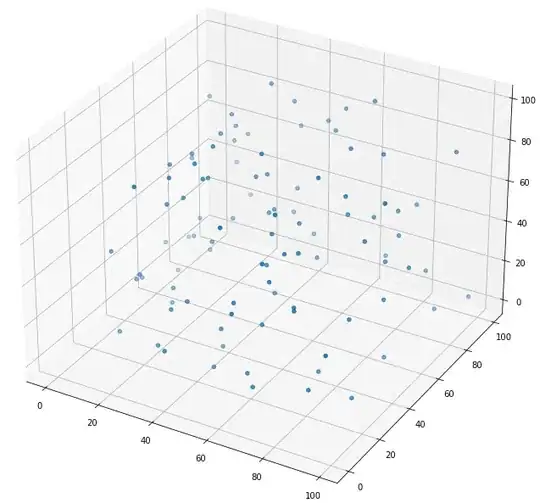