I think what you need to use lookarounds in your case:
(?<!\p{Alnum})test(?!\p{Alnum})
The negative lookbehind (?<!\p{Alnum})
will fail the match if there is an alphanumeric char present to the left of the test
, and the negative lookahead (?!\p{Alnum})
will fail the match if there is an alphanumeric char right after test
.
See the testing screenshot:
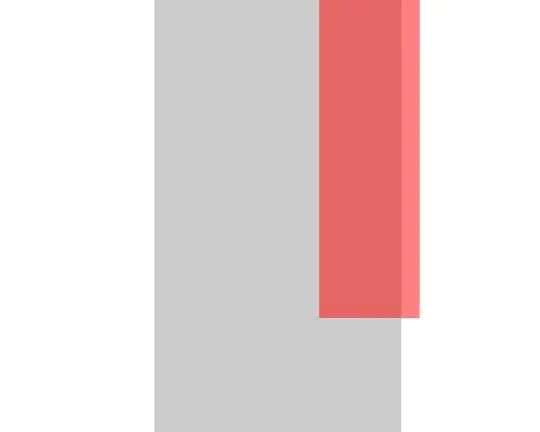
Java demo:
String str = "this is#test_ :";
Pattern ptrn = Pattern.compile("(?<!\\p{Alnum})test(?!\\p{Alnum})");
Matcher matcher = ptrn.matcher(str);
while (matcher.find()) {
System.out.println(matcher.start());
}
Alternative way: match and capture the search word, and print the start position of the 1st capturing group:
Pattern ptrn = Pattern.compile("\\P{Alnum}(test)\\P{Alnum}");
...
System.out.println(matcher.start(1));
See this Java demo
NOTE that in this scenario, the \P{Alnum}
is a consuming pattern, and in some edge cases, test
might not get matched.