I'm not aware of your patterns but this is how I typically implement DDS
using System;
using System.Linq;
using EPiServer.Data;
using EPiServer.Data.Dynamic;
using EPiServer.ServiceLocation;
namespace Herlitz.EPiBlog.Models.DDS
{
public interface IDailyXMasLotteryWinner
{
DailyXMasLotteryWinner Create(string fullName);
DailyXMasLotteryWinner Get(string fullName);
bool Exist(string property, string value);
}
[ServiceConfiguration(typeof(IDailyXMasLotteryWinner))]
public class DailyXMasLotteryWinner : IDailyXMasLotteryWinner //,IDynamicData
{
private readonly DynamicDataStore _store = DynamicDataStoreFactory.Instance.CreateStore(typeof(DailyXMasLotteryWinner));
public Identity Id { get; set; }
public string FullName { get; set; }
public DailyXMasLotteryWinner Create(string fullName)
{
if (Exist(property: "FullName", value: fullName))
{
return Get(fullName: fullName);
}
Id = Identity.NewIdentity(Guid.NewGuid());
FullName = fullName;
_store.Save(this);
return this;
}
public DailyXMasLotteryWinner Get(string fullName)
{
return _store.Find<DailyXMasLotteryWinner>(propertyName: "FullName", value: fullName).FirstOrDefault();
}
public bool Exist(string property, string value)
{
return _store.Find<DailyXMasLotteryWinner>(propertyName: property, value: value).Any();
}
}
}
Tested with this
@{
var xmasLocator = ServiceLocator.Current.GetInstance<IDailyXMasLotteryWinner>();
var winner = xmasLocator.Create(fullName: "Ned Flanders");
<div>@winner.Id @winner.FullName</div>
}
And it works
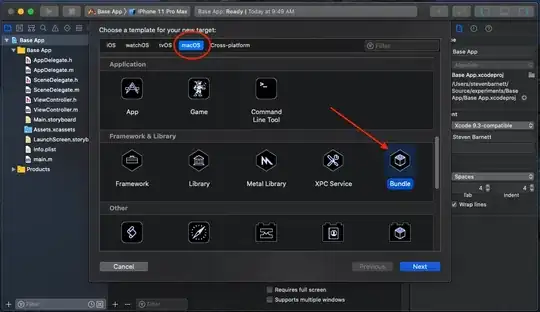
Obviously you should adapt this to your patterns, if it doesn't work it would probably be your database user that has insufficient rights.