For the record, the code can be written as simply
public static boolean isLeapYearGregorian(int year) {
return (year % 4 == 0) && (year % 100 != 0) || (year % 400 == 0);
}
But for the sake of starting close to the OP's code, let's begin with this:
public static boolean isLeapYearGregorian(int year) {
return (year % 4 == 0) && (year % 100 != 0) || (year % 4 == 0) && (year % 100 == 0) && (year % 400 == 0);
}
At this point, you need to know the operator precedence in Java, because the ands (&&
) and ors (||
) above are at the same level, and it makes a difference. Short answer: AND comes before OR.
Now the logic can be listed as:
- (year % 4 == 0) AND (year % 100 != 0)
- OR
- (year % 4 == 0) AND (year % 100 == 0) AND (year % 400 == 0)
Which is to say:
- (year divisible by 4) AND (year not divisible by 100)
- OR
- (year divisible by 4) AND (year divisible by 100) AND (year divisible by 400)
Now you can compare the logic with the pseudocode of the definition of a Gregorian calendar leap year:
if (year is not divisible by 4) then (it is a common year)
else if (year is not divisible by 100) then (it is a leap year)
else if (year is not divisible by 400) then (it is a common year)
else (it is a leap year)
From here it is straightforward to compare to the logic we extracted from the code in your question, and verify that yes the code correctly identifies leap years, as defined by Wikipedia.
If the comparison doesn't seem all that "straightforward", you can resort to truth tables, and prove exhaustively that the two logical statements are the same.
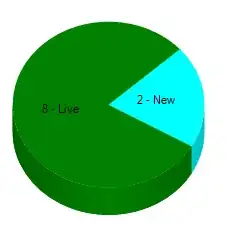