It's good practice to name the class Song
instead of Songs
since it will represent only one song.
With adding songs manually to the listBox
private List<Song> SongList;
public Form1()
{
InitializeComponent();
SongList = new List<Song>();
}
private void button1_Click(object sender, EventArgs e)
{
Song song = new Song(newTracktextBox.Text, 100);
SongList.Add(song);
listBox1.Items.Add(song); // The trackName will be shown because we are doing a override on the ToString() in the Song class
}
class Song
{
private string trackName;
private int trackLength;
public Song(string trackName, int trackLength)
{
this.trackName = trackName;
this.trackLength = trackLength;
}
public override string ToString()
{
return trackName;
// Case you want to show more...
// return trackName + ": " + trackLength;
}
}
With automatic binding by using a BindingList<Song>
private BindingList<Song> SongList;
public Form1()
{
InitializeComponent();
// Initialise a new list and bind it to the listbox
SongList = new BindingList<Song>();
listBox1.DataSource = SongList;
}
private void button1_Click(object sender, EventArgs e)
{
// Create a new song and add it to the list,
// the listbox will automatically update accordingly
Song song = new Song(newTracktextBox.Text, 100);
SongList.Add(song);
}
class Song
{
private string trackName;
private int trackLength;
public Song(string trackName, int trackLength)
{
this.trackName = trackName;
this.trackLength = trackLength;
}
public override string ToString()
{
return trackName;
}
}
Result
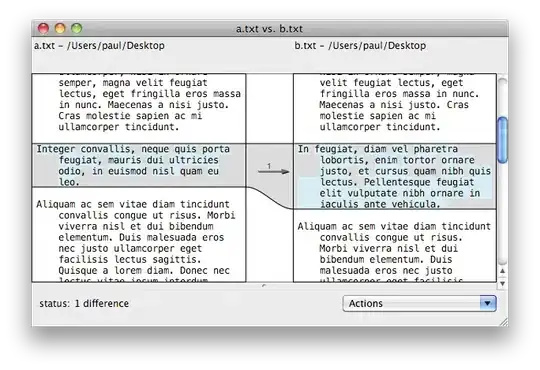