Maybe there is an another approach for achieving this, but mine is as following:-
1) Create Trigger on Table After Insert.
This Trigger is responsible for executing a job.
2) Create a SQL Server Agent job Via T-SQL.
This a Job is responsible for Updating the table after one hour.
The Complete Demo: {Tested also}
This Demo is Updating The Table after one Minute.
Create database DBTest
go
use DBTest
go
Create table TblTransaction (CreateDate datetime,
TransactionNo int,
Status varchar (10))
go
USE msdb
go
Create procedure [dbo].[sp_add_job_quick]
@job nvarchar(128),
@mycommand nvarchar(max),
@servername nvarchar(28),
@startdate nvarchar(8),
@starttime nvarchar(8)
as
--Add a job
EXEC dbo.sp_add_job
@job_name = @job ;
--Add a job step named process step. This step runs the stored procedure
EXEC sp_add_jobstep
@job_name = @job,
@step_name = N'Update Status After one Hour',
@subsystem = N'TSQL',
@command = @mycommand
--Schedule the job at a specified date and time
exec sp_add_jobschedule @job_name = @job,
@name = 'MySchedule',
@freq_type=1,
@active_start_date = @startdate,
@active_start_time = @starttime
-- Add the job to the SQL Server Server
EXEC dbo.sp_add_jobserver
@job_name = @job,
@server_name = @servername
go
use DBTest
go
Create Trigger trg_UpdateRecordAfterOneHour on TblTransaction
After insert
as
declare @JobName varchar (100),
@TransactionNo int,
@mycommand varchar (512),
@startdate varchar (100),
@startTime varchar(100)
select @TransactionNo = TransactionNo from inserted
set @JobName = 'MyJob_' + convert(nvarchar(MAX), GETDATE(), 113)
set @mycommand = 'Update DBTest..TblTransaction set status = ''PAID'' where TransactionNo =' + convert(varchar(100), @TransactionNo)
set @startdate = convert(nvarchar(MAX), GETDATE(), 112)
set @starttime = Replace(convert(varchar(10), dateadd(MINUTE,1,GETDATE()), 108),':','')
exec msdb.dbo.sp_add_job_quick
@JobName ,
@mycommand,
@@servername,
@startdate,
@starttime
Let's Insert an Record , Then Select this Record.
insert TblTransaction values (getdate(),1,'UnPaid')
select * from TblTransaction
Result:
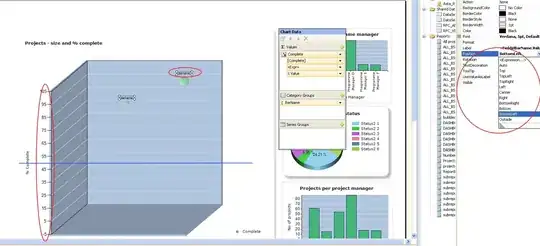
Select Again after one minute.
select * from TblTransaction
Result:
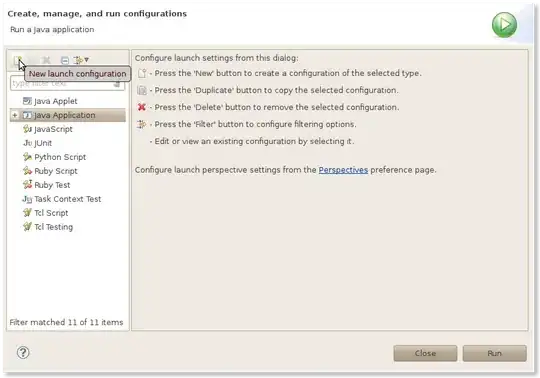
Quick Notes:
1) Type HOUR
instead of MINUTE
for executing the job after one hour rather than one minute.
2) The functionality of creating SQL Agent Jobs is not available in SQL Server Express Edition, it is available for Enterprise , Business Intelligence, Standard & Web editions.
3) By default, the SQL Server Agent service is disabled when SQL Server is installed unless the user explicitly chooses to autostart the service.
Update according to a below comment:-
4) The Job that Created is working for a once, according the demo while creating [dbo].[sp_add_job_quick]
, I passed 1
for @freq_type
as following:
@freq_type=1
The accepted values for @freq_type is as following:
╔═══════╦════════════════════════════════════════╗
║ Value ║ Description ║
╠═══════╬════════════════════════════════════════╣
║ 1 ║ Once ║
║ 4 ║ Daily ║
║ 8 ║ Weekly ║
║ 16 ║ Monthly ║
║ 32 ║ Monthly, relative to freq_interval ║
║ 64 ║ Run when SQLServerAgent service starts ║
║ 128 ║ Run when the computer is idle ║
╚═══════╩════════════════════════════════════════╝
for more details sp_add_schedule (Transact-SQL):