I ) Try GIDSignIn Class for Authentication :
II ) import required classes as fallows :
#import <UIKit/UIKit.h>
#import <GoogleSignIn/GoogleSignIn.h>
@interface ViewController : UIViewController<GIDSignInDelegate,GIDSignInUIDelegate,NSURLSessionDelegate>
@end
III) in viewDidLoad do as fallows:
- (void)viewDidLoad {
[super viewDidLoad];
[GIDSignIn sharedInstance].delegate = self;
[GIDSignIn sharedInstance].uiDelegate = self;
[GIDSignIn sharedInstance].clientID = "your_CLIENT_ID; //get from console
[[GIDSignIn sharedInstance] setScopes:@[@"https://www.googleapis.com/auth/plus.login",@"https://www.googleapis.com/auth/plus.me",@"https://www.googleapis.com/auth/contacts",@"https://www.googleapis.com/auth/contacts.readonly"]];
// Button for opening Google Auth //
GIDSignInButton *button = [[GIDSignInButton alloc]initWithFrame:CGRectMake(0.0f, 0.0f, 200.0f, 200.0f)];
button.style = kGIDSignInButtonStyleWide;
button.colorScheme =kGIDSignInButtonColorSchemeLight;
[button setCenter:self.view.center];
[self.view addSubview:button];
}

IV ) as soon as the user finishes sign in handle it as below by GIDSignInUIDelegate
:
- (void)signIn:(GIDSignIn *)signIn didSignInForUser:(GIDGoogleUser *)user withError:(NSError *)error {
// Perform any operations on signed in user here.
NSString *userId = user.userID; // For client-side use only!
NSString *idToken = user.authentication.idToken; // Safe to send to the server
NSString *fullName = user.profile.name;
NSString *givenName = user.profile.givenName;
NSString *familyName = user.profile.familyName;
NSString *email = user.profile.email;
[self getPeopleList];
// ...
}
// Implement these methods only if the GIDSignInUIDelegate is not a subclass of
// UIViewController.
// Stop the UIActivityIndicatorView animation that was started when the user
// pressed the Sign In button
- (void)signInWillDispatch:(GIDSignIn *)signIn error:(NSError *)error {
NSLog(@"0.0.");
}
// Present a view that prompts the user to sign in with Google
- (void)signIn:(GIDSignIn *)signIn presentViewController:(UIViewController *)viewController {
[self presentViewController:viewController animated:YES completion:nil];
}
// Dismiss the "Sign in with Google" view
- (void)signIn:(GIDSignIn *)signIn dismissViewController:(UIViewController *)viewController {
[self dismissViewControllerAnimated:YES completion:nil];
}
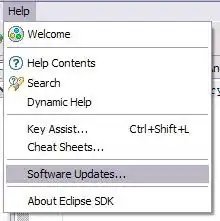
V ) now in -(void)getPeopleList{}
you will find contacts :
-(void)getPeopleList{
NSString *url = [NSString stringWithFormat:@"https://people.googleapis.com/v1/people/me/connections?personFields=emailAddresses"];
NSURL *linkurl = [NSURL URLWithString:url];
NSURLSessionConfiguration *configuration = [NSURLSessionConfiguration defaultSessionConfiguration];
NSURLSession *session = [NSURLSession sessionWithConfiguration:configuration delegate:self delegateQueue:nil];
NSMutableURLRequest *request = [NSMutableURLRequest requestWithURL:linkurl
cachePolicy:NSURLRequestUseProtocolCachePolicy
timeoutInterval:60.0];
[request addValue:@"application/json" forHTTPHeaderField:@"Accept"];
[request addValue:[NSString stringWithFormat:@"Bearer %@",[GIDSignIn sharedInstance].currentUser.authentication.accessToken] forHTTPHeaderField:@"Authorization"];
[request setHTTPMethod:@"GET"];
NSURLSessionDataTask *postDataTask = [session dataTaskWithRequest:request completionHandler:^(NSData *data, NSURLResponse *response, NSError *error) {
if (!error) {
NSDictionary *user_contact_json = [NSJSONSerialization JSONObjectWithData:data options:0 error:nil];
NSLog(@"user_contacts %@", user_contact_json);
} else {
NSLog(@"Error %@",error);
}
}];
[postDataTask resume];
}
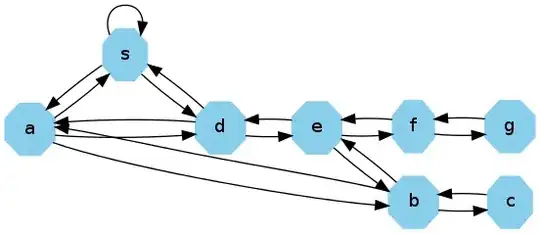