Start with:
Make sure the <div id="content">
is loaded to DOM before you 'set text' script executes, could utilize onload()
to ensure. Probably checked this, but a heads up for others.
Next, I wonder if the issue is with the .html()
method of JQuery. Documentation states "This method uses the browser's innerHTML property."
Check innerHTML()
alone via:
document.getElementById("content").innerHTML = "test";
I know there are certain limitation when utilizing innerHTML()
in vanilla JS, for instance issues with <script>
, so if JQuery's .html()
utilizes it, it may be an issue somehow.
If you can, try using vanilla JS to set the #content
<div>
via:
document.getElementById("content").textContent = "test";
This will allow you eliminate .html()
and it's use of .innerHTML()
truly isn't to blame.
Edit: Here is JQuery's .html()
method, the true issue may lie with how it handles the setting. It attempts to use innerHTML()
, if that fails somehow, it then defaults to append()
.
See below:
function (value) {
return access(this, function (value) {
var elem = this[0] || {},
i = 0,
l = this.length;
if (value === undefined && elem.nodeType === 1) {
return elem.innerHTML;
}
// See if we can take a shortcut and just use innerHTML
if (typeof value === "string" && !rnoInnerhtml.test(value) && !wrapMap[(rtagName.exec(value) || ["", ""])[1].toLowerCase()]) {
value = value.replace(rxhtmlTag, "<$1></$2>");
try {
for (; i < l; i++) {
elem = this[i] || {};
// Remove element nodes and prevent memory leaks
if (elem.nodeType === 1) {
jQuery.cleanData(getAll(elem, false));
elem.innerHTML = value;
}
}
elem = 0;
// If using innerHTML throws an exception, use the fallback method
} catch(e) {}
}
if (elem) {
this.empty().append(value);
}
},
null, value, arguments.length);
}
Furthermore, here is the source for the fallback default called, append()
, if the innerHTML()
in the html()
method fails:
function () {
return this.domManip(arguments, function (elem) {
if (this.nodeType === 1 || this.nodeType === 11 || this.nodeType === 9) {
var target = manipulationTarget(this, elem);
target.appendChild(elem);
}
});
}
You can find the methods in JQuery by searching with Ctrl + F and searching when viewing the source... for instance html:
, with the colon. See picture below:
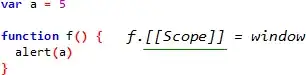
Notice the search bar on the bottom.