it is very simple. you have to create a file called file.php in that file you place following code:
<?php
session_start();
if (isset($_GET["name"]) && isset($_GET["email"]) && !empty($_GET["name"]) && !empty($_GET["email"])) {
if(isset($_SESSION['view']))
{
$_SESSION['view']=$_SESSION['view']+1;
}
else
{
$_SESSION['view']=1;
}
$myfile = fopen("submissions.html", "a") or die("Unable to open file!");
$line = "*** ". $_SESSION["view"] . " ***";
$date = "Submitted: " . date("Y/m/d H:i", time());
$name = $_GET["name"];
$email = $_GET["email"];
$line2 = "";
fwrite($myfile, "\n". $line);
fwrite($myfile, "\n". $date);
fwrite($myfile, "\n". $name);
fwrite($myfile, "\n". $email);
fwrite($myfile, "\n". $line2);
fclose($myfile);
} else {
header('Location: index.html');
}
?>
and in the index.html this content:
<html>
<head>
<title>Form</title>
</head>
<body>
<form method="get" action="file.php">
<label>Name</label>
<input title="name" required name="name" type="text" />
<label>Email</label>
<input title="email" required name="email" type="email" />
<input type="submit" value="Submit">
</form>
</body>
</html>
for explanaition:
this code will count for the current session. its required for
*** (int)+1 ***
if(isset($_SESSION['view']))
{
$_SESSION['view']=$_SESSION['view']+1;
}
else
{
$_SESSION['view']=1;
}
here you open the file. a
stands for append
:
$myfile = fopen("submissions.html", "a") or die("Unable to open file!");
this code will format your output:
$line = "*** ". $_SESSION["view"] . " ***";
$date = "Submitted: " . date("Y/m/d H:i", time());
$name = $_GET["name"];
$email = $_GET["email"];
$line2 = "";
and will look like:
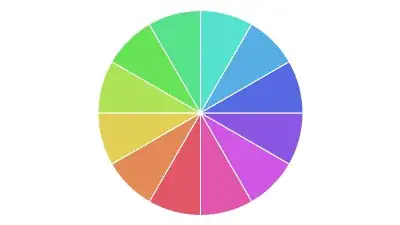
then we write every line:
fwrite($myfile, "\n". $line);
fwrite($myfile, "\n". $date);
fwrite($myfile, "\n". $name);
fwrite($myfile, "\n". $email);
fwrite($myfile, "\n". $line2);
fclose($myfile);