I am going to explain it in some simple way and I hope it will useful for you.
1) Create empty Console application.
2) Make a service reference to any public OData service. I.e. http://services.odata.org/northwind/northwind.svc/
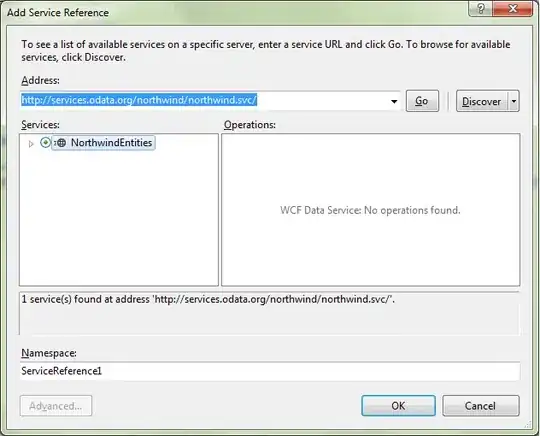
After this Visual Studio is going to add some more assembly references like you can see below
3) Write the following code
using System; using System.Collections.Generic; using
System.Data.Services.Client; using System.Linq; using System.Text;
using System.Threading.Tasks;
namespace ConsoleApplication4
{
class Program
{
static DataServiceContext ctx = new DataServiceContext(new Uri("http://services.odata.org/northwind/northwind.svc/"));
static void Main(string[] args)
{
IEnumerable<ServiceReference1.Category> response =
ctx.Execute<ServiceReference1.Category>(new Uri("http://services.odata.org/northwind/northwind.svc/Categories"));
}
}
}
4) Setup a breakpoint at the end of the Main method. And now debug application.
You are going to see the list of Categories.
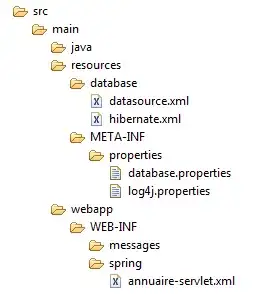
5) If the OData have exposed with permission to realize all CRUD then you can do it.
And surely you can return response
in ASP .NET MVC but first you have to transform it into your Model class.
Perhaps you can keep static DataServiceContext ctx = new DataServiceContext(new Uri("http://services.odata.org/northwind/northwind.svc/"));
in your BaseController class.
And also you get property value like this:
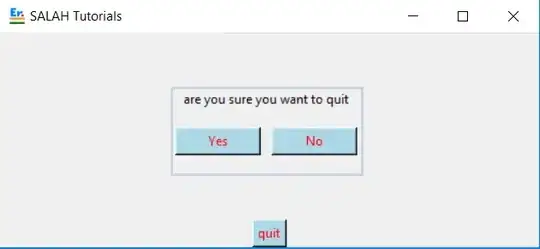
P.S. Take a look at this video http://www.youtube.com/watch?v=e07TzkQyops as well.