Go with this demo, Shared Demo
After the demo, If you still face any problem then let me know.
Now Listen Here
I think you need output something like this,
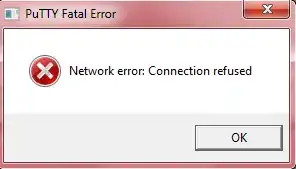
Follow the steps: -
Create a new viewcontroller(says, CustomTableVC) in your storyboard and one UITableView(give constraints and delegate to its own class), take outlet of UItableView (says, tblMyCustom)
Now press CLT+N for new file and do like this below image, Subclass - UItableViewCell and also tick on XIB option.
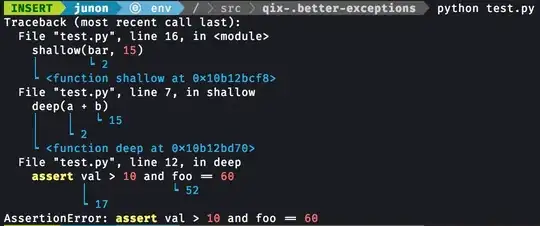
Open our xib file, add new UIView (says myView, as you see highted in below image), in this myView add two labels
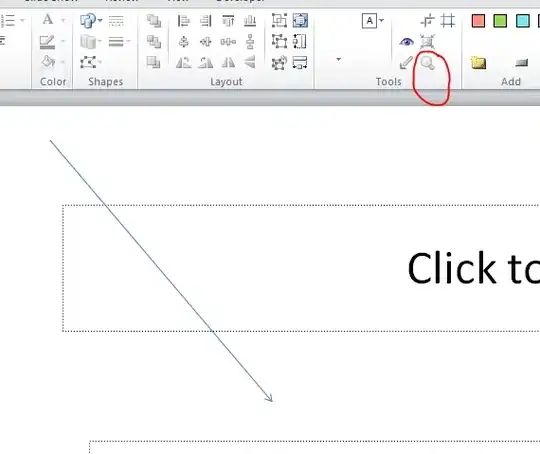
Now take outlet of these two labels in its customCell class
class CustomTableCell: UITableViewCell {
@IBOutlet var lblLeaderNo: UILabel!
@IBOutlet var lblDistict: UILabel!
override func awakeFromNib() {
super.awakeFromNib()
// Initialization code
}
override func setSelected(_ selected: Bool, animated: Bool) {
super.setSelected(selected, animated: animated)
// Configure the view for the selected state
}
}
Now back to your Viewcontroller Class
import UIKit
class CustomTableVC: UIViewController , UITableViewDelegate, UITableViewDataSource{
@IBOutlet var tblMyCustom: UITableView!
var leaderno : [String]!
var distict : [String]!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view.
self.tblMyCustom.register(UINib(nibName: "CustomTableCell", bundle: nil), forCellReuseIdentifier: "customCell")
self.leaderno = ["1", "2", "3", "4"]
self.distict = ["Delhi","Kerala", "Haryana", "Punjab"]
// above both array must have same count otherwise, label text in null
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
public func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int{
return leaderno.count;
}
public func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell{
var customCell: CustomTableCell! = tableView.dequeueReusableCell(withIdentifier: "customCell") as? CustomTableCell
customCell.lblLeaderNo.text = self.leaderno[indexPath.row]
customCell.lblDistict.text = self.distict[indexPath.row]
return customCell
}
}
above all is code of VC, it is not getting settle down here in single code format, I dont know why.
Now, follow these steps you get output as i show you image in starting of the procedure.