Make the data frame.
df <- data.frame(idplaces = c("201220","201321","201422","201525"),
Amex = c(0,1,0,1),
visa = c(1,1,01,1),
mastercard = c(0,1,0,1),
cash = c(1,1,1,1))
First, you have to reformat the data (that's often the case for ggplot2). The way to reformat it depends on how you intend to plot it. I'll be using geom_bar()
, which defaults to having the height of the bars represent the number of cases in the data frame. So I'll reformat to a long data frame using gather
from the tidyr package, and then filter out any cases where the restaurant doesn't accept that method. The resulting dataframe will have columns for the restuarant id, and one row for each of the payment methods each restaurant accepts.
> df_long <- df %>%
+ gather("method", "accepts", -idplaces, factor_key = TRUE) %>%
+ filter(accepts == 1)
> df_long
idplaces method accepts
1 201321 Amex 1
2 201525 Amex 1
3 201220 visa 1
4 201321 visa 1
5 201422 visa 1
6 201525 visa 1
7 201321 mastercard 1
8 201525 mastercard 1
9 201220 cash 1
10 201321 cash 1
11 201422 cash 1
12 201525 cash 1
To plot with methods on the x-axis, set x=method
in aes()
.
> ggplot(df_long, aes(x=method)) +
+ geom_bar()
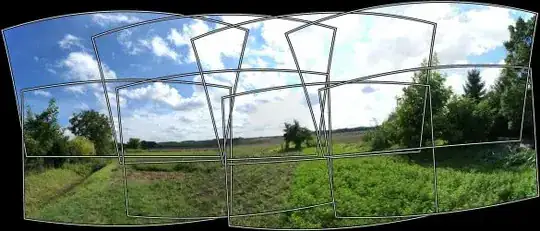