Pandas DataFrames, such as pandoc
, have a plot
method with a kind
parameter.
So it is possible to make a plot using
pandoc.plot(x='date_time', y='total_goals', kind="bar", ax=tg)
Notice that ax=tg
is used to tell pandoc
to draw on the matplotlib Axes, tg
.
In contrast, matplotlib Axes, such as tg
, have a plot
method, but tg.plot
does not have a kind
parameter. Instead, to make a bar plot with an Axes object, call its tg.bar
method.
Using the pandoc.plot
method, you could make a bar plot using something like
import numpy as np
import matplotlib.pyplot as plt
import pandas as pd
np.random.seed(2016)
N = 150
pandoc = pd.DataFrame({'date_time':pd.date_range('2000-1-1', periods=N, freq='M'),
'total_goals':np.random.randint(10, size=N)})
fig, tg = plt.subplots(1)
pandoc.plot(x='date_time', y='total_goals', kind="bar", ax=tg)
labels, skip = ['']*N, 10
labels[skip//2::skip] = pandoc['date_time'].dt.strftime('%Y-%m-%d')[skip//2::skip]
tg.set_xticklabels(labels)
fig.autofmt_xdate()
plt.show()
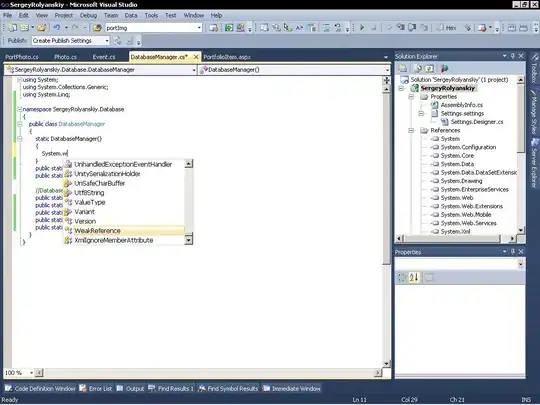
Note that tg.set_xticklabels
is used to set the xticklabels instead of mdates.DateFormatter
. When making a bar plot the underlying bar plot xtick
values are integers:
In [21]: tg.get_xticks()
Out[26]: array([0, 1, 2, 3, 4, 5, 6, 7, 8, 9])
You can only use mdates.DateFormatter
when the xtick
values are dates.
Since a bar plot has a fixed number of bars, there is no advantage to using a dynamic formatter like mticker.FuncFormatter
; you are best off simply setting the xticklabels
using the Axes.set_xticklabels
method.
labels = ['']*N
creates a list of N
empty strings. For example, ['']*2
evaluates to ['', '']
.
x, y = a, b
is equivalent to
x = a
y = b
So labels, skip = ['']*N, 10
is equivalent to
labels = ['']*N
skip = 10
Python slice notation, e.g. x[start:end:step]
is explained here. For example,
In [227]: x = list('ABCDEFGHIJK'); x
Out[227]: ['A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J', 'K']
In [228]: x[1::3]
Out[228]: ['B', 'E', 'H', 'K'] <-- the first item is x[1], and then we pick up every 3rd item
So in the code above, pandoc['date_time'].dt.strftime('%Y-%m-%d')
is a sequence of strings and if we call it x
, then x[skip//2::skip]
is a new sequence which starts with x[skip//2]
and then steps by skip
amount.
skip//2
divides skip
by 2 using integer-division.
labels
begins as a list of N
empty strings. With skip=10
, the assignment
labels[skip//2::skip] = pandoc['date_time'].dt.strftime('%Y-%m-%d')[skip//2::skip]
replaces every 10th element (starting at skip//2
) with a date string from pandoc['date_time'].dt.strftime('%Y-%m-%d')
.
pandoc['date_time']
is a time series.
pandoc['date_time'].dt.strftime('%Y-%m-%d')
uses the Series.dt.strftime
method to format the dates into date-strings in %Y-%m-%d
format.