Understand it using the below example
Weight defines how much space a view will consume compared to other views within a LinearLayout
.
Weight is used when you want to give specific screen space to one component compared to other.
Key Properties:
weightSum
is the overall sum of weights of all child views. If you don't specify the weightSum
, the system will calculate the sum of all the weights on its own.
layout_weight
specifies the amount of space out of the total weight sum
the widget will occupy.
Code:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/activity_main"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="horizontal"
android:weightSum="4">
<EditText
android:layout_weight="2"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:text="Type Your Text Here" />
<Button
android:layout_weight="1"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:text="Text1" />
<Button
android:layout_weight="1"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:text="Text1" />
</LinearLayout>
The output is:
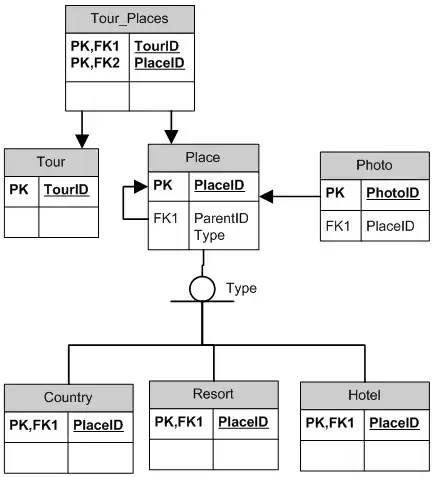
Now even if the size of the device is larger, the EditText will take 2/4 of the screen's space. Hence the look of your app is seen consistent across all screens.
[This if before you edit your question might be irrelevant now
Now in your Bonfire at the beach there is no weight and its wrap_content
so there is no grantee that it will take the remaining space! and that space can remain after adding it will differ with the screen size of device ]
Note:
Here the layout_width
is kept 0dp
as the widget space is divided horizontally. If the widgets are to be aligned vertically layout_height
will be set to 0dp
.
This is done to increase the efficiency of the code because at runtime the system won't attempt to calculate the width or height respectively as this is managed by the weight. If you instead used wrap_content
the system would attempt to calculate the width/height first before applying the weight attribute which causes another calculation cycle.
Lets Move to your XML
see how i used them
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="match_parent"
android:weightSum="3" //<-------------------
android:layout_height="match_parent">
<ImageView
android:src="@drawable/mc"
android:layout_width="match_parent"
android:layout_height="0dp"
android:scaleType="centerCrop"
android:layout_weight = "1"/> //<-------------------
<TextView
android:text="You're invited!"
android:layout_width="match_parent"
android:layout_height="0dp"
android:textColor="@android:color/white"
android:textSize="54sp"
android:layout_weight = "1" //<-------------------
android:background="#009688" />
<TextView
android:layout_weight = "1" //<------------------- if you remove this , this text view will be gone cuz its 0 by default
android:text="Bonfire at the beach"
android:layout_width="match_parent"
android:layout_height="0dp"
android:textColor="@android:color/white"
android:textSize="34sp"
android:background="#009688" />
</LinearLayout>
Now you ask what if you have not given android:layout_weight
,Default weight is zero. Zero means view will not be shown, that empty space will remain there
Since you don't believe you can read the documentation
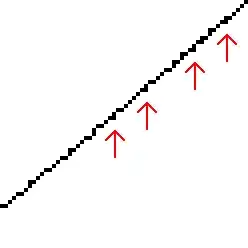
EDIT: 2
Since you said that, i went through android-visualizer that you use
and Have you ever noticed this...
"Line 6: The attribute android:weight_sum
is not supported here."
thing on its bottom.
Meaning they are not providing that functionality to adjust your layout boundaries. Its just a simple online tool.I am
not saying it is not recommended to use, but my personal idea is, you
can't touch the depth of android if you use that.
Now if you want a confirmation what actually happens have a look on android studio/ eclipse as well which are read IDE s
This is android studio
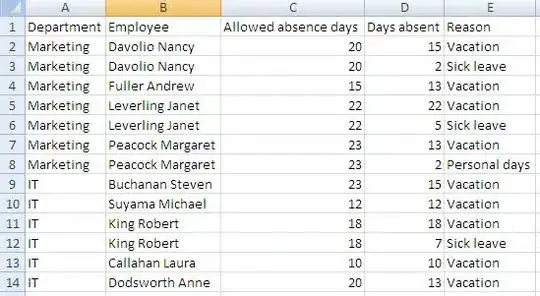
Can you see any view contain your text "Bonfire at the beach"? no
Instead a.studio display a red line in XML.
Suspicious size: this will make the view invisible
Because there is no layout_weight
is given and we have added 0 height
Now you can accept the answer :)