You’ve been using the process.stdout writable stream implicitly every time you’ve called console.log()
. Internally, the console.log()
function calls process.stdout.write()
after formatting the input arguments. But the console functions are more for debugging and inspecting objects. When you need to write structured data to stdout, you can call process.stdout.write()
directly.
Say your program connects to an HTTP URL and writes the response to stdout. Stream#pipe()
works well in this context, as shown here:
var http = require('http');
var url = require('url');
var target = url.parse(process.argv[2]);
var req = http.get(target, function (res) {
res.pipe(process.stdout);
});
Before you can read from stdin, you must call process.stdin.resume()
to indicate that your script is interested in data from stdin. After that, stdin acts like any other readable stream, emitting data events as data is received from the output of another process, or as the user enters keystrokes into the terminal window.
The following listing shows a command-line program that prompts the user for their age before deciding whether to continue executing.
To improve @Joseph's answer, you can use both command in between back ticks() and
$(enter code here)` :
$ node --arg1=`echo 1` --arg2=$(echo 2)
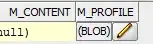