You can use a combination of horizontal and vertical stack views to achieve this:
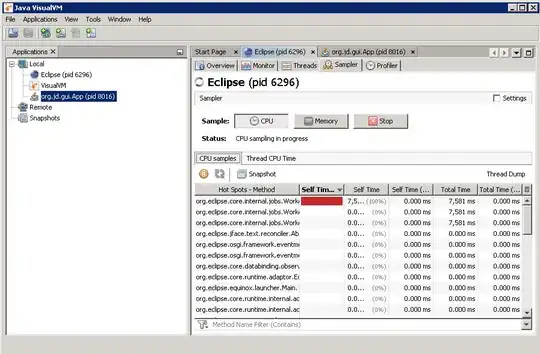
This solution allows you to avoid two pitfalls with the other approaches suggested above:
- You don't have to mess with toggling views' hidden property
- You aren't limited to a fixed maximum number of items
Here's the code:
import UIKit
class Order {
var stations = [Station]()
var companies = [String]()
}
class Station {
let name: String
let amount: Int
init(name: String, amount: Int) {
self.name = name
self.amount = amount
}
}
class TableViewController: UITableViewController {
var orders = [Order]()
override func viewDidLoad() {
super.viewDidLoad()
tableView!.rowHeight = UITableViewAutomaticDimension
tableView!.estimatedRowHeight = 44.0
//simulated data
let order1 = Order()
order1.companies.append("Global @ CITGO Braintree, Braintree")
order1.companies.append("Exxon @ Global, Chelsea")
order1.stations.append(Station(name: "Tyngsboro Mobil #2871", amount: 4000))
order1.stations.append(Station(name: "Grafton St #10095", amount: 15000))
orders.append(order1)
let order2 = Order()
order2.companies.append("Exxon @ Global, Chelsea")
order2.stations.append(Station(name: "Winstead Citgo #2001", amount: 3000))
orders.append(order2)
let order3 = Order()
order3.companies.append("MSCG @ Sunoco Partners")
order3.stations.append(Station(name: "Citgo #123", amount: 7000))
order3.stations.append(Station(name: "Mobil #345", amount: 12500))
order3.stations.append(Station(name: "Exxon #567", amount: 300))
order3.stations.append(Station(name: "Citgo #789", amount: 6100))
order3.stations.append(Station(name: "Stoughton Mobil #2744", amount: 9098))
order3.stations.append(Station(name: "Westborough Mobil #2720", amount: 120000))
orders.append(order3)
}
override func numberOfSections(in tableView: UITableView) -> Int {
return 1
}
override func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return orders.count
}
override func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "MyTableViewCell", for: indexPath) as! TableViewCell
let order = orders[indexPath.row]
cell.companyLabel.text = ""
for (index, company) in order.companies.enumerated() {
cell.companyLabel.text! += company
if index < order.companies.count - 1 {
cell.companyLabel.text! += "\n"
}
}
for station in order.stations {
// create horizontal stack view to arrange the station name and amount
let horizontalStackView = UIStackView()
horizontalStackView.axis = .horizontal
let nameLabel = UILabel()
nameLabel.translatesAutoresizingMaskIntoConstraints = false
nameLabel.text = station.name
horizontalStackView.addArrangedSubview(nameLabel)
let amountLabel = UILabel()
amountLabel.translatesAutoresizingMaskIntoConstraints = false
amountLabel.addConstraint(NSLayoutConstraint(item: amountLabel, attribute: .width, relatedBy: .equal, toItem: nil, attribute: .width, multiplier: 1.0, constant: 60))
amountLabel.backgroundColor = UIColor.purple
amountLabel.textAlignment = .right
amountLabel.textColor = UIColor.white
amountLabel.text = String(station.amount)
horizontalStackView.addArrangedSubview(amountLabel)
// add the horizontal stack view to the vertical stack view containing all stations
cell.stackView.addArrangedSubview(horizontalStackView)
}
return cell
}
}
Here's what the storyboard looks like:
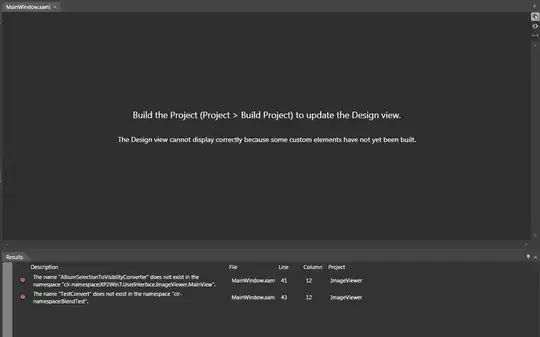