How about a customized Toast instead? One that is far more alluring, suits your need and requires no libraries or complex implications?
Now let us try the following bit of code
func sailAwayLabelAction(){
// here creating a rectangle with certain dimensions you can easily manipulate
let rect = CGRect(origin: CGPoint(x: self.view.frame.size.width/2 - 150,y :self.view.frame.size.height-100), size: CGSize(width: 300, height: 35))
//here creating and manipulating the attributes of your text, i.e color,alignment etc..
let toastLabel = UILabel(frame: rect)
toastLabel.backgroundColor = UIColor.orange
toastLabel.textColor = UIColor.white
toastLabel.textAlignment = NSTextAlignment.center;
toastLabel.text = "This is my customized Toast !"
toastLabel.layer.cornerRadius = 10;
toastLabel.clipsToBounds = true
//first pop the toast into our view
self.view.addSubview(toastLabel)
//then after 1 sec + 1 sec delay, animate the entire toastLabel out.
UIView.animate(withDuration: 1, delay: 1, usingSpringWithDamping: 1, initialSpringVelocity: 1, options: .curveEaseOut, animations: {
toastLabel.alpha = 0.0
})
}
Whenever you activate the previous function, it should render something similar to this,
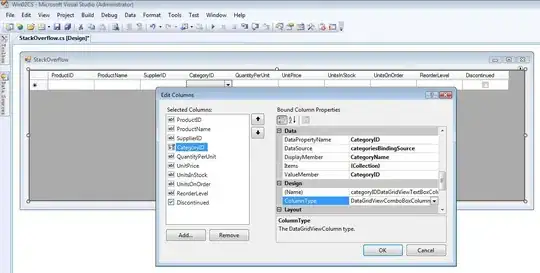