You can upload the image asynchronously using a JavaScript function. Because you included the jQuery tag, here is an example with jQuery. It uses jQuery.ajax() to upload the file, append() to add the newly uploaded image to the page.
var list = document.getElementById('demo');
function changeText2() {
var firstname = document.getElementById('firstname').value;
document.getElementById('boldStuff2').innerHTML = firstname;
var entry = document.createElement('li');
entry.appendChild(document.createTextNode(firstname));
list.appendChild(entry);
var formData = new FormData($('form')[0]);
$.ajax({
url: '/museum/uploadImage.php', //Server script to process data
type: 'POST',
//Ajax events
success: completeHandler,
// Form data
data: formData,
//Options to tell jQuery not to process data or worry about content-type.
cache: false,
contentType: false,
processData: false
});
}
function completeHandler(data) {
if (data.result) {
if (data.result[0] == '/') {
var newImage = $('<img>',{
src: data.result
});
var closeLink = $('<span>', {class: "closeImage"});
var container = $('<span>').append(closeLink, newImage);
$('#uploadedImages').append(container);
}
else {
$('#errorMsg').html(data.result);
}
}
}
I applied those functions to the sample in this answer and connected it with the PHP code below to handle uploading the image and returning the path to the image. See it in action on my site. It uses preg_match() to ensure the uploaded file is an image file (by checking the MIME-Type) with an image extension (adjust accordingly) and move_uploaded_file() to handle moving the temporary file to a path to store uploaded files.
<?php
$uploads_dir = '/upload_dir';
$output = array();
if (is_array($_FILES) && array_key_exists('image',$_FILES)) {
$upload = $_FILES['image'];
if ($upload['error'] == UPLOAD_ERR_OK &&
preg_match('#^image\/(png|jpg|jpeg|gif)$#',$upload['type']) && //ensure mime-type is image
preg_match('#.(png|jpg|jpeg|gif)$#',$upload['name']) //ensure name ends in trusted extension
) {
$tmp_name = $upload["tmp_name"];
// basename() may prevent filesystem traversal attacks;
// further validation/sanitation of the filename may be appropriate
$parts = explode('/',$upload['tmp_name']);
$tmpName = array_pop($parts);
$name = $tmpName.'_'.basename($upload["name"]);
if (move_uploaded_file($tmp_name, "$uploads_dir/$name")) {
$output['result'] = '/museum/'.$name;
}
else {
$output['result'] = 'upload fail';
}
}
else {
$output['result'] = 'invalid image';
}
}
else {
$output['result'] = 'no file selected';
}
header('Content-type: application/json');
echo json_encode($output);
?>
After selecting an image and clicking the Submit button, the image is uploaded and the uploaded image is displayed with the image to close it.
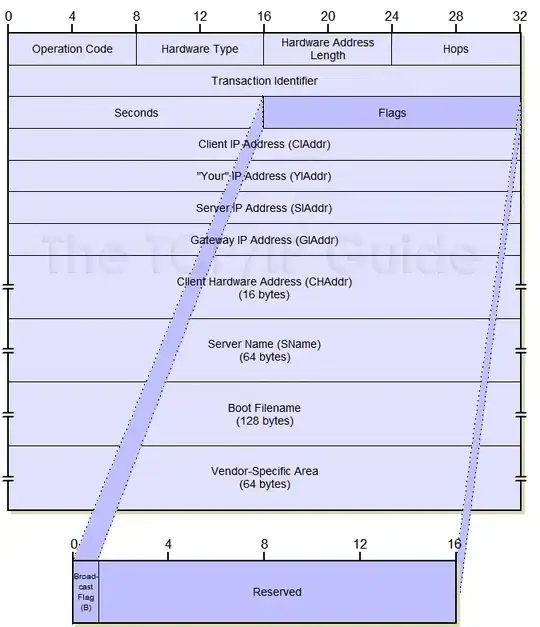
For the close links, add an Event delegate like the one in the javascript below:
$(document).ready(function(readyEvent) {
$(document).click(function(clickEvent) {
if ($(clickEvent.target).hasClass('closeImage')) {
clickEvent.target.parentNode.remove();
}
});
});
.closeImage {
position: absolute;
float: left;
clear: none;
width: 15px;
height: 14px;
background: url(https://www.secretsof.com/images/close_x.png) no-repeat center center;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div id="uploadedImages"><span><span class="closeImage"></span>
<img src="https://i.stack.imgur.com/J15os.png">
</span>
</div>