You need to specify an ItemTemplate
for the ListBox
to use for each item. Then within that template one needs to have a TextBlock
which has its property TextWrapping
set to Wrap
.
But real the problem, which is solved below, is that one runs into a situation where when first drawn the TextBlock
will expand to the needed space. But after that it will not resize.
By binding to a parent which can resize (note that not all things do not resize automatically) we can get such a change.
One thing I like to do during the design phase is to give the items being styled specific colors to determine how things are drawn.
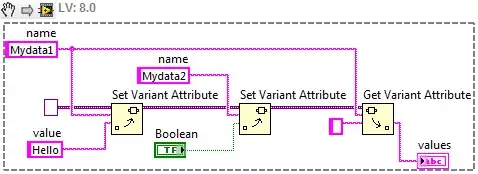
Code
<Grid Background="DarkGreen">
<ListBox x:Name="lbChatHistory"
ItemsSource="{StaticResource Orders}"
HorizontalAlignment="Stretch"
VerticalAlignment="Stretch"
Background="DarkBlue"
Margin="10">
<ListBox.ItemsPanel>
<ItemsPanelTemplate>
<VirtualizingStackPanel VerticalAlignment="Bottom" />
</ItemsPanelTemplate>
</ListBox.ItemsPanel>
<ListBox.ItemTemplate>
<DataTemplate>
<TextBlock Text="{Binding CustomerName, FallbackValue=Unknown}"
FontSize="14"
Foreground="White"
TextWrapping="Wrap"
Width="{Binding ElementName=lbChatHistory, Path=ActualWidth}"
VerticalAlignment="Stretch"
HorizontalAlignment="Stretch"
Background="DarkRed"/>
</DataTemplate>
</ListBox.ItemTemplate>
</ListBox>
</Grid>
For this example completeness the control is bound to a list of Orders
with obvious properties, but your data will differ of course.
<Page.Resources>
<model:Orders x:Key="Orders">
<model:Order CustomerName="Alpha"
OrderId="997"
InProgress="True" />
<model:Order CustomerName="Beta"
OrderId="998"
InProgress="False" />
<model:Order CustomerName="Omega"
OrderId="999"
InProgress="True" />
<model:Order CustomerName="The rain in spain falls mainly"
OrderId="1000"
InProgress="False" />
</model:Orders>
</Page.Resources>
This answer is similar to an answer I gave ListBoxItem HorizontalContentAlignment To Stretch Across Full Width of ListBox which may be needed for your next question as you color the surrounding textblock.