Showing the data one after the other seems a bit unergonomic to me. Also using an animation might not be the best solution. What if after inspection of the second dataset you want to go back to the first?
I would therefore implement a solution which allows to go back and forth between the spectra.
The following sandbox example is based on a solution I have provided to a similar problem with images. It uses a discrete Slider to walk through the pages. Although it may seem a bit complicated on first sight, you do not really have to understand the PageSlider
class in order to use it. Just look at the code down in the __main__
part.
import matplotlib.widgets
import matplotlib.patches
import mpl_toolkits.axes_grid1
class PageSlider(matplotlib.widgets.Slider):
def __init__(self, ax, label, numpages = 10, valinit=0, valfmt='%1d',
closedmin=True, closedmax=True,
dragging=True, **kwargs):
self.facecolor=kwargs.get('facecolor',"w")
self.activecolor = kwargs.pop('activecolor',"b")
self.fontsize = kwargs.pop('fontsize', 10)
self.numpages = numpages
super(PageSlider, self).__init__(ax, label, 0, numpages,
valinit=valinit, valfmt=valfmt, **kwargs)
self.poly.set_visible(False)
self.vline.set_visible(False)
self.pageRects = []
for i in range(numpages):
facecolor = self.activecolor if i==valinit else self.facecolor
r = matplotlib.patches.Rectangle((float(i)/numpages, 0), 1./numpages, 1,
transform=ax.transAxes, facecolor=facecolor)
ax.add_artist(r)
self.pageRects.append(r)
ax.text(float(i)/numpages+0.5/numpages, 0.5, str(i+1),
ha="center", va="center", transform=ax.transAxes,
fontsize=self.fontsize)
self.valtext.set_visible(False)
divider = mpl_toolkits.axes_grid1.make_axes_locatable(ax)
bax = divider.append_axes("right", size="5%", pad=0.05)
fax = divider.append_axes("right", size="5%", pad=0.05)
self.button_back = matplotlib.widgets.Button(bax, label=ur'$\u25C0$',
color=self.facecolor, hovercolor=self.activecolor)
self.button_forward = matplotlib.widgets.Button(fax, label=ur'$\u25B6$',
color=self.facecolor, hovercolor=self.activecolor)
self.button_back.label.set_fontsize(self.fontsize)
self.button_forward.label.set_fontsize(self.fontsize)
self.button_back.on_clicked(self.backward)
self.button_forward.on_clicked(self.forward)
def _update(self, event):
super(PageSlider, self)._update(event)
i = int(self.val)
if i >=self.valmax:
return
self._colorize(i)
def _colorize(self, i):
for j in range(self.numpages):
self.pageRects[j].set_facecolor(self.facecolor)
self.pageRects[i].set_facecolor(self.activecolor)
def forward(self, event):
current_i = int(self.val)
i = current_i+1
if (i < self.valmin) or (i >= self.valmax):
return
self.set_val(i)
self._colorize(i)
def backward(self, event):
current_i = int(self.val)
i = current_i-1
if (i < self.valmin) or (i >= self.valmax):
return
self.set_val(i)
self._colorize(i)
if __name__ == "__main__":
import numpy as np
from matplotlib import pyplot as plt
num_pages = 10
data = np.random.rand(700, num_pages)
spec = np.linspace(-10,10, 700)
fig, ax = plt.subplots()
fig.subplots_adjust(bottom=0.18)
ax.set_ylim([0.,1.6])
line, = ax.plot(spec,data[:,0], color="b")
ax_slider = fig.add_axes([0.1, 0.05, 0.8, 0.04])
slider = PageSlider(ax_slider, 'Page', num_pages, activecolor="orange")
def update(val):
i = int(slider.val)
line.set_ydata(data[:,i])
slider.on_changed(update)
plt.show()
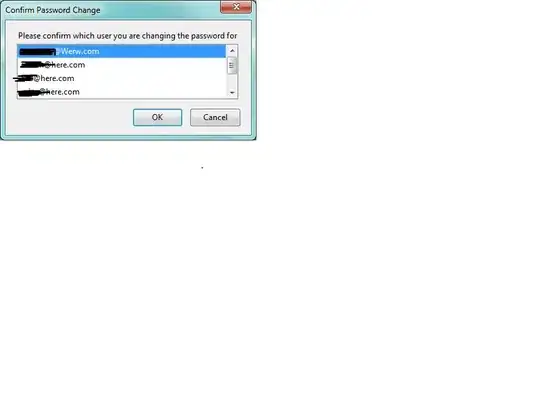
The code above is working and shows how this would look like. In your specific case, you would need to change it a bit.
I tried to adapt your code accordingly, but of course I cannot guarantee that it works. This code has to be put below the __main__
part, the PageSlider must stay unchanged.
import numpy as np
from matplotlib import pyplot as plt
dateien = ['./2450ATT0.csv','./2450ATT0-1.csv','./2450ATT0-2.csv']
data_x = []
data_y = []
for datei in dateien: #do not call a variable "file" in python as this is protected
x = np.genfromtxt(datei, usecols =(0), delimiter=';', unpack=True)
x = x/1000000.
y = np.genfromtxt(datei, usecols =(1), delimiter=';', unpack=True, dtype=float)
data_x.append(x)
data_y.append(y)
fig, ax = plt.subplots()
fig.subplots_adjust(bottom=0.18)
ax.set_xlim([2435,2465])
ax.set_xlim([-120,20])
ax.set_xlabel('Frequenz')
ax.set_ylabel('Leistung')
text = ax.text(0.98,0.98, dateien[0], ha="right", va="top")
line, = ax.plot(data_x[0],data_y[0], color="b")
ax_slider = fig.add_axes([0.1, 0.05, 0.8, 0.04])
slider = PageSlider(ax_slider, 'Page', len(dateien), activecolor="orange")
def update(val):
i = int(slider.val)
line.set_data(data_x[i],data_y[i])
text.set_text(dateien[i])
slider.on_changed(update)
plt.show()
Edit:
For a simple animation, you would rather use matplotlib.animation.FuncAnimation
and the code would look something along those lines
import numpy as np
from matplotlib import pyplot as plt
dateien = ['./2450ATT0.csv','./2450ATT0-1.csv','./2450ATT0-2.csv']
data_x = []
data_y = []
for datei in dateien: # do not call a variable "file" in python, this is a protected word
x = np.genfromtxt(datei, usecols =(0), delimiter=';', unpack=True)
x = x/1000000.
y = np.genfromtxt(datei, usecols =(1), delimiter=';', unpack=True, dtype=float)
data_x.append(x)
data_y.append(y)
fig, ax = plt.subplots()
fig.subplots_adjust(bottom=0.18)
ax.set_xlim([2435,2465])
ax.set_xlim([-120,20])
ax.set_xlabel('Frequenz')
ax.set_ylabel('Leistung')
line, = ax.plot(data_x[0],data_y[0], color="b")
def update(i):
line.set_data(data_x[i],data_y[i])
ani = matplotlib.animation.FuncAnimation(fig, update,
frames= len(dateien), interval = 200, blit = False, repeat= True)
plt.show()