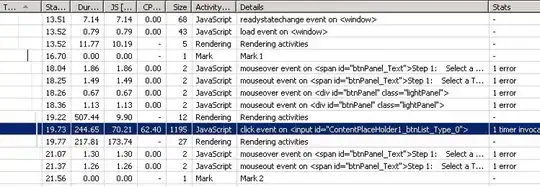
It would be more clear to imagine that p
points to the whole memory block of 5 int
. So if there is a ( p + 1 )
it would point to the next memory block.
This then help answer your questions.
How could p equals *p?
p
is pointer to array[5] that is initialized to point to arr[5]
*p
is the value of the first array element - which is the address of arr[5]
. And because the name of the array is the address of the first element. So when you print p
and *p
they are the same.
And now that p equals to &arr, why *p != arr[0]?
*p
is the address of the first array element - which means the address of the whole arr[5]
memory block. On the other hand, arr[0]
is the first element of the array that p
points to, so it's a real value (1) not an address.
What exactly does the value p and *p hold?
As your first question, both of them hold the address of the whole arr[5]
memory block.