Too broad as answer, but had this from old blog:
taking_screenshot_in_android.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/layout"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:layout_margin="16dp"
android:gravity="center"
android:orientation="vertical">
<Button
android:id="@+id/capture_screen_shot"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:onClick="screenShot"
android:text="Take ScreenShot" />
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="5dp"
android:text="" />
<ImageView
android:id="@+id/imageView"
android:layout_width="200dp"
android:layout_height="250dp"
android:background="#ccc"
android:padding="5dp" />
<TextView
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:autoLink="web"
android:gravity="center|bottom"
android:text="ViralAndroid.com"
android:textSize="26sp"
android:textStyle="bold" />
</LinearLayout>
TakingScreenShotAndroid.java
import android.graphics.Bitmap;
import android.os.Bundle;
import android.os.Environment;
import android.support.v7.app.AppCompatActivity;
import android.view.View;
import android.widget.Button;
import android.widget.ImageView;
import android.widget.TextView;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.FileOutputStream;
public class TakingScreenShotAndroid extends AppCompatActivity {
TextView textView;
ImageView imageView;
Bitmap mbitmap;
Button captureScreenShot;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.taking_screenshot_in_android);
textView = (TextView) findViewById(R.id.textView);
textView.setText("Your ScreenShot Image:");
captureScreenShot = (Button) findViewById(R.id.capture_screen_shot);
imageView = (ImageView) findViewById(R.id.imageView);
}
public void screenShot(View view) {
mbitmap = getBitmapOFRootView(captureScreenShot);
imageView.setImageBitmap(mbitmap);
createImage(mbitmap);
}
public Bitmap getBitmapOFRootView(View v) {
View rootview = v.getRootView();
rootview.setDrawingCacheEnabled(true);
Bitmap bitmap1 = rootview.getDrawingCache();
return bitmap1;
}
public void createImage(Bitmap bmp) {
ByteArrayOutputStream bytes = new ByteArrayOutputStream();
bmp.compress(Bitmap.CompressFormat.JPEG, 40, bytes);
File file = new File(Environment.getExternalStorageDirectory() +
"/capturedscreenandroid.jpg");
try {
file.createNewFile();
FileOutputStream outputStream = new FileOutputStream(file);
outputStream.write(bytes.toByteArray());
outputStream.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
strings.xml
<string name="app_name">Taking Screenshot in Android Programmatically</string>
Output:
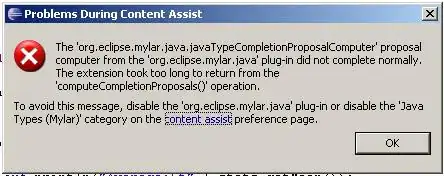