Yes, use localStorage.
When using persisted values, you'll need to check to see if a value was already saved (from the user's previous visit) and, if so, use that. If not, you'll need to create a value and set it into storage so that it can be used on a subsequent visit.
This is generally done like this:
var x = null;
// Check to see if there is already a value in localStorage
if(localStorage.getItem("x")){
// Value exists. Get it assign it:
x = localStorage.getItem("x");
} else {
// Value doesn't exist. Set it locally and store it for future visits
x = 3.14;
x = Math.floor(x);
localStorage.setItem("x", x);
}
In your example, you have a variety of issues, but the browser locking up was due to the while
loop, which was creating an infinite loop whenever the life count was not zero. You must be VERY careful with while
loops and ensure that they will always have a termination condition that will be hit. But, for your purposes, this was unnecessary anyway.
See comments inline below for details on the correct usage of localStorage
.
NOTE: This code won't work here in the Stack Overflow code snippet editor due to sandboxing, but you can see this working example here.
// This is where you will store the lives remaining
// You don't need several variables to keep track of this
// (mainLife, life, life1). Just modify this one variable as needed.
var lifeCount = null;
// As soon as the user arrives at the page, get or set the life count
window.addEventListener("DOMContentLoaded", initLifeCount);
// By convention use camelCase for identifier names that aren't constructors
// and put opening curly brace on same line as declaration
function initLifeCount() {
// You need to test to see if an item exists in localStorage before getting it
if(localStorage.getItem("life")){
// And, remember that localStorage values are stored as strings, so you must
// convert them to numbers before doing math with them.
lifeCount = parseInt(localStorage.getItem("life"), 10) - 1;
// Test to see if there are any lives left
if(lifeCount === 0){
// Invoke "Game Over" code
alert("Game Over!");
}
} else {
// User hasn't previously stored a count, so they must be starting a new game
lifeCount = 3;
}
// Any time the count changes, remember to update it in localStorage
updateLifeCount();
// Temporary code for debugging:
console.log("Life count is: " + lifeCount);
}
// Call this function after the life count changes during game play
function updateLifeCount(){
localStorage.setItem("life", lifeCount)
}
If you open your developer tools (F12) and navigate to view the localStorage
, you will be able to see the value decrease every time you re-run the Fiddle. You can also see the value in the console.
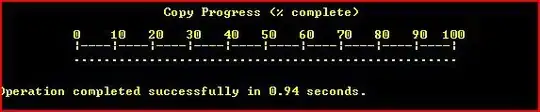
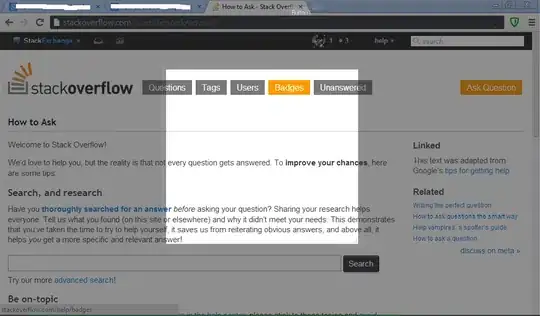