The family of xxxMap()
operators all deal with higher-order Observables. Which means they allow you to create Observables inside the main Observable and inline the resulting values into the primary stream. So you could read the type signature as Observable<Observable<T>> => Observable<T>
Given a stream which every emission x is an Observable containing 4 value emissions:
input: --x----------x
flatMap a-a-a-a-| b-b-b-b-|
result: --a-a-a-a----b-b-b-b-|
Typecasting xxxMap(myFnc) return values
The xxxMap()
operators all work with results of type Observable
, Promise
or Array
. Depending on what you put into it will get converted to Observable if needed.
Rx.Observable.of('')
.flatMap(() => [1,2,3,4])
.subscribe(val => console.log('array value: ' + val));
Rx.Observable.of('')
.flatMap(() => Promise.resolve(1))
.subscribe(val => console.log('promise value: ' + val));
Rx.Observable.of('')
.flatMap(() => Promise.resolve([1,2,3,4]))
.subscribe(val => console.log('promise array value: ' + val));
Rx.Observable.of('')
.flatMap(() => Rx.Observable.from([1,2,3,4]))
.subscribe(val => console.log('Observable value: ' + val));
<script src="https://cdnjs.cloudflare.com/ajax/libs/rxjs/5.0.3/Rx.js"></script>
In your case you can easily flatMap the objects and return the arrays:
Rx.Observable.of({ error: null, alternatives: ['result1', 'result2', 'result3'] })
.flatMap(val => val.alternatives)
.subscribe(console.log);
<script src="https://cdnjs.cloudflare.com/ajax/libs/rxjs/5.0.3/Rx.js"></script>
Difference between all xxxMap operators
What does mergeMap do
flatMap
, better known as mergeMap
will merge
all emissions whenever they come into the main stream.
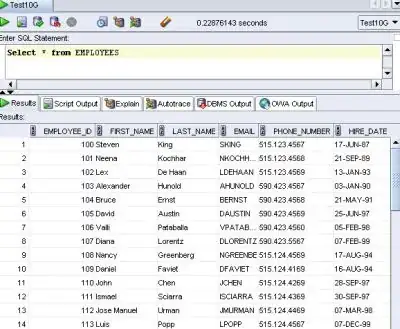
concatMap
concatMap will wait for all emissions to complete before concat
the next stream:
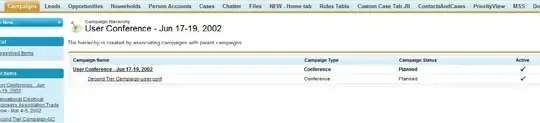
switchMap
but switchMap will abandon the previous stream when a new emission is available and switch to emit values from the new stream:
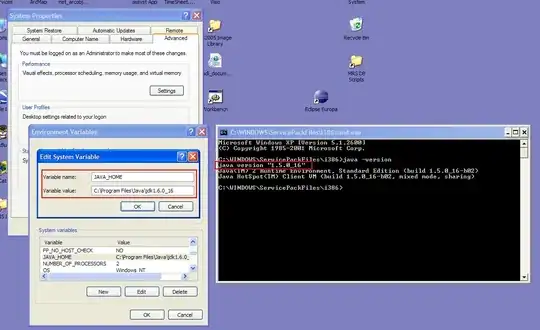