Just posting this for posterity as accepted answer deserves credit, but if anyone finds themselves in a similar predicament, here is a snippet of the code that worked for me:
// my injected code
window.addEventListener('load', () => {
const injectDiv = document.createElement('div')
const shadowRoot = injectDiv.attachShadow({ mode: 'open' })
// note inline use of webpack raw-loader, so that the css
// file gets inserted as raw text, instead of attached to <head>
// as with the webpack style-loader
shadowRoot.innerHTML = // just using template string
`
<style>${require('raw-loader!app/styles/extension-material.css')}</style>
<div id='shadowReactRoot' />
`
document.body.appendChild(injectDiv)
ReactDOM.render(
<App />,
// note you have to start your query in the shadow DOM
// in order to find your root
shadowRoot.querySelector('#shadowReactRoot')
)
})
Then, sure enough:
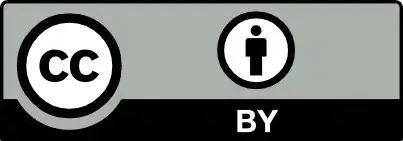