TlDr: android:gravity Works with RelativeLayout , you can skip to the bottom if you don't want to read the explanation
EDIT this is a giant wall of text, please bear with me
This is what I imagine you want to achieve:
<TextView
android:id="@+id/title"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textAppearance="@style/TextAppearance.AppCompat.Title"
android:textAlignment="center"
android:text="@string/app_title"
android:layout_marginBottom="@dimen/main_spacing"
android:textSize="24sp"
android:layout_centerHorizontal="true"/> <!-- LAYOUT PARAMS WORK!!!-->
Why does this work, while android:gravity
(apparently) doesn't?
if your goal is to put the TextView in the horizontal center of the Layout/Screen you have to let the RelativeLayout
know!
this is achieved through something called LayoutParams
- these are data structures defined by each View
which are used by the View's parent (in this case the RelativeLayout)
so let's say your TextView
has the following LayoutParams
:
android:layout_centerHorizontal="true"
you will get something like this:
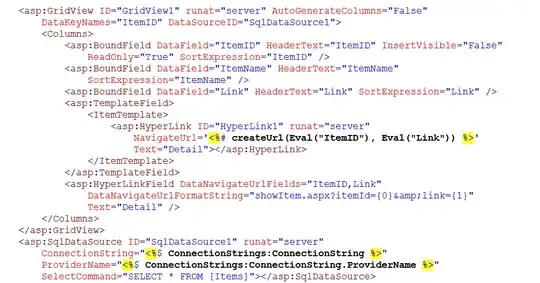
When the RelativeLayout
is distributing its child views around the screen, along the way there is a callback method being run - onLayout(...) - which is part of a more complex method sequence that will determine the position of each child View inside the RelativeLayout, this is achieved in part by accessing the LayoutParams
in each child View
, in this case that line in your TextView
- This is why we say LayoutParams are passed on to the parent like in the link you mentioned before
WARNING: Endless Confusion Source!
View positioning inside Layout
s / ViewGroup
s is done through LayoutParam
s the onLayout
calls
It so happens that some layouts like FrameLayout
have a LayoutParam called android:layout_gravity which causes great confusion with the android:gravity
property that each view can define, which is NOT the same and not even a LayoutParam
android:gravity
is used by any View (like a TextView
for example) to place its content inside.
Example: let's say that you change your TextView to be very high, and you want the text at the bottom of the TextView, instead of at the top
<TextView
android:id="@+id/title"
android:layout_width="wrap_content"
android:layout_height="95dp" <------------ very high!
android:textAppearance="@style/TextAppearance.AppCompat.Title"
android:textAlignment="center"
android:text="@string/app_title"
android:layout_marginBottom="@dimen/main_spacing"
android:textSize="24sp"
android:layout_centerHorizontal="true" <----- LayoutParams for RelativeLayout
/>
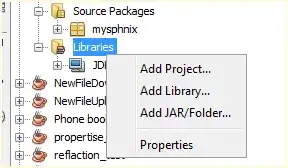
TextView is CENTERED in the RelativeLayout but text is at the TOP of the "box"
Let's use android:gravity to manage the text position INSIDE THE TextView
<TextView
android:id="@+id/title"
android:layout_width="wrap_content"
android:layout_height="95dp"
android:textAppearance="@style/TextAppearance.AppCompat.Title"
android:textAlignment="center"
android:text="@string/app_title"
android:layout_marginBottom="@dimen/main_spacing"
android:textSize="24sp"
android:layout_centerHorizontal="true"
android:gravity="bottom" <---**NOT LayoutParams**, NOT passed to RLayout
/>
Result: As expected only the inside of the View changed
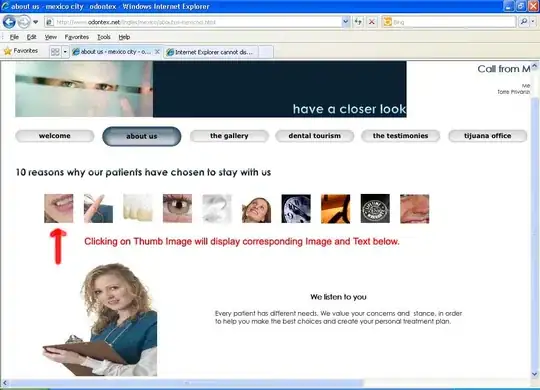
TLDR
Now if you want to ignore everything above and still use android:gravity with RelativeLayout, RelativeLayout
is also a View
so it has the android:gravity property, but you have to remove other properties or LayoutParams which will override the behaviour defined by the android:gravity property look at android:gravity at work with RelativeLayout
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/activity_main"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
android:gravity="bottom"> <!-- NOT LAYOUT PARAMS -->
<TextView
android:id="@+id/title"
android:layout_width="wrap_content"
android:layout_height="95dp"
android:textAppearance="@style/TextAppearance.AppCompat.Title"
android:textAlignment="center"
android:text="@string/app_title"
android:layout_marginBottom="@dimen/main_spacing"
android:textSize="24sp"
android:layout_centerHorizontal="true"
/>
<AutoCompleteTextView
android:id="@+id/enterContact"
android:text="Enter Contact"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textAlignment="center"
android:layout_below="@+id/title"
android:layout_marginBottom="@dimen/main_spacing" />
</RelativeLayout>
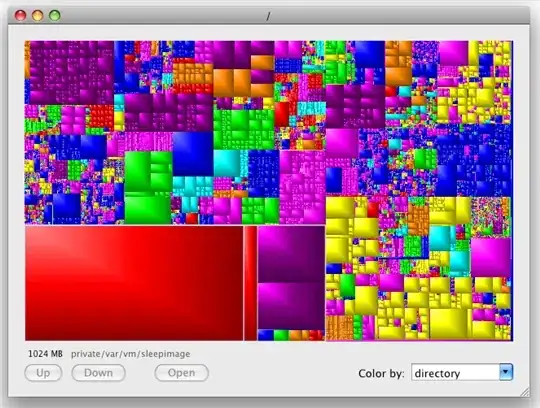