Sounds like a fun exercise, so here is my take on this:
Please note that is by no means production-ready code, but rather a quick demonstration of the idea behind this. Also the snippet below is written in the context of a playground in order to test things quickly.
import UIKit
import PlaygroundSupport
let label = UILabel(frame: CGRect(x: 0, y: 0, width: 120, height: 30))
let text = "marquee effect on UILabel by removing letters in front and adding in end in swift 3"
label.lineBreakMode = .byClipping
label.text = text
label.backgroundColor = .white
label.textAlignment = .left
let timer = Timer.scheduledTimer(withTimeInterval: 0.3, repeats: true) { _ in
DispatchQueue.main.async {
if let text = label.text, !text.isEmpty {
let index = text.index(after: text.startIndex)
label.text = label.text?.substring(from: index)
}
}
}
PlaygroundPage.current.liveView = label
Which gets you this:
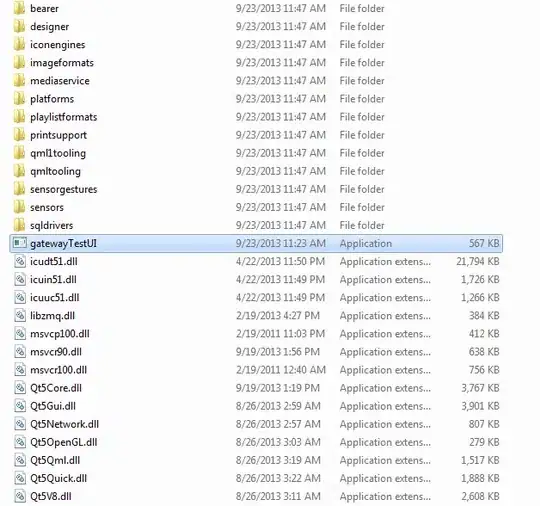
Some notes on this:
- You can use some other mechanism instead of a timer such as a
CADisplayLink
or a custom animation callback
- The effect would work better with a monospaced font
- This code doesn't handle a reset when the text is empty or the timer invalidates
- The effect works by setting the whole text in the label, set its clipping mode as to display only characters that are inside the bounds of it & finally removing characters from the beginning of the string. I do not see a real reason to do the remove/insert 'dance' that you are describing in the question but this would be something trivial to do...
anyway, I hope that this makes sense :)