July, 2023 Update:
You can extend User model with OneToOneField() to add extra fields. *You can also see my answer explaining how to set up email
and password
authentication with AbstractUser or AbstractBaseUser and PermissionsMixin.
First, run the command below to create account
app:
python manage.py startapp account
Then, set account
app to INSTALLED_APPS in settings.py
as shown below:
# "settings.py"
INSTALLED_APPS = [
...
"account", # Here
]
Then, create UserProfile
model in account/models.py
as shown below. *user
field extends User
model with OneToOneField()
:
# "account/models.py"
from django.db import models
from django.utils.translation import gettext_lazy as _
from django.contrib.auth.models import User
class UserProfile(models.Model):
user = models.OneToOneField(
User,
verbose_name=_("user"),
on_delete=models.CASCADE
)
age = models.PositiveSmallIntegerField(_("age"))
gender = models.CharField(_("gender"), max_length=20)
married = models.BooleanField(_("married"))
Then, create UserProfileInline
and UserAdmin
classes in account/admin.py
as shown below. *With admin.site.unregister(User)
, unregistering User
model registered by default is necessary otherwise there is error:
# "account/admin.py"
from django.contrib import admin
from .models import UserProfile
from django.contrib.auth.admin import UserAdmin as BaseUserAdmin
from django.contrib.auth.models import User
admin.site.unregister(User) # Necessary
class UserProfileInline(admin.TabularInline):
model = UserProfile
@admin.register(User)
class UserAdmin(BaseUserAdmin):
inlines = (UserProfileInline,)
Then, run the command below:
python manage.py makemigrations && python manage.py migrate
Then, run the command below:
python manage.py runserver 0.0.0.0:8000
Finally, you could extend User
model with extra fields as shown below:
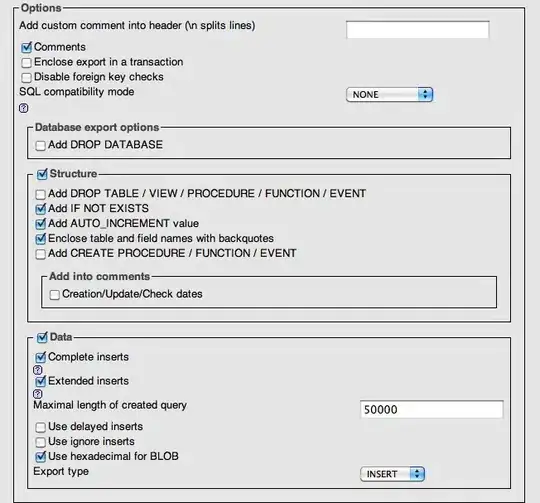