I have done your job, as you don't know. Please follow my code below and do changes what you needed in your application. But you should include library files hosted by your server for faster results::
FULL CODE
<link href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" rel="stylesheet">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.1.1/jquery.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script>
<script type="text/javascript">
var data = [
{
"UserID": 1,
"UserName": "rooter",
"Password": "12345",
"Country": "UK",
"Email": "sac@gmail.com"
},
{
"UserID": 2,
"UserName": "binu",
"Password": "123",
"Country": "uk",
"Email": "Binu@gmail.com"
},
{
"UserID": 3,
"UserName": "cal",
"Password": "123",
"Country": "uk",
"Email": "cal@gmail.com"
},
{
"UserID": 4,
"UserName": "nera",
"Password": "1234",
"Country": "uk",
"Email": "nera@gmail.com"
}
];
$(document).ready(function () {
var html = '<table class="table table-striped">';
html += '<tr>';
var flag = 0;
$.each(data[0], function(index, value){
html += '<th>'+index+'</th>';
});
html += '</tr>';
$.each(data, function(index, value){
html += '<tr>';
$.each(value, function(index2, value2){
html += '<td>'+value2+'</td>';
});
html += '<tr>';
});
html += '</table>';
$('body').html(html);
});
</script>
AND it will look like image below:
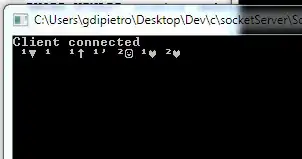