First, get the data into a data.frame:
data <- data.frame(BOROUGH = c("BRONX", "BROOKLYN", "MANHATTAN", "QUEENS", "STATEN ISLAND"),
tpedinjury = c(3975, 8781, 6607, 5893, 653),
tcycinjury = c(959, 3978, 3164, 1993, 101),
tminjury = c(11197, 25822, 8698, 21092, 3528))
Here's what that looks like:
> data
BOROUGH tpedinjury tcycinjury tminjury
1 BRONX 3975 959 11197
2 BROOKLYN 8781 3978 25822
3 MANHATTAN 6607 3164 8698
4 QUEENS 5893 1993 21092
5 STATEN ISLAND 653 101 3528
For plotting ease, we'll want it in long format. I like to use gather()
from the tidyr
package for that, but there are several other functions that also do the same thing, so take your pick.
data <- gather(data, "injurytype", "number", -BOROUGH)
Now the data look like this:
> data
BOROUGH injurytype number
1 BRONX tpedinjury 3975
2 BROOKLYN tpedinjury 8781
3 MANHATTAN tpedinjury 6607
4 QUEENS tpedinjury 5893
5 STATEN ISLAND tpedinjury 653
6 BRONX tcycinjury 959
7 BROOKLYN tcycinjury 3978
8 MANHATTAN tcycinjury 3164
9 QUEENS tcycinjury 1993
10 STATEN ISLAND tcycinjury 101
11 BRONX tminjury 11197
12 BROOKLYN tminjury 25822
13 MANHATTAN tminjury 8698
14 QUEENS tminjury 21092
15 STATEN ISLAND tminjury 3528
Use geom_bar(stat = "identity")
with fill = injurytype
to get a stacked bar plot.
ggplot(data, aes(x=BOROUGH, y=number, fill = injurytype)) +
geom_bar(stat = "identity")
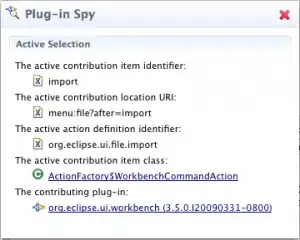