You can parse you data with Regex! It's marvelous!
There're plenty (Left
, Mid
, Right
, Instr
) functions to parse your data, right?
Some people, when confronted with a problem, think
“I know, I'll use regular expressions.”
Now they have two problems.
I think, that you trying to bound to some keywords(tagstr, text and ttl), so take a look at this.
Feel free to modify this expression, take a look on this and that!
In VBA there's no regex from scratch , so add VBA reference to "Microsoft VBScript Regular Expressions 5.5"

This is my exapmle with your data:
Sub test()
Dim Data As String
Dim Re As RegExp
Dim ReMatch As MatchCollection
Dim CurrentMatch As Match
Data = "[{" & Chr(34) & "q" & Chr(34) & ":{" & Chr(34) & "as" & Chr(34) & ":[{" & Chr(34) & "id" & Chr(34) & ":" & Chr(34) & "1" & Chr(34) & "," & Chr(34) & "tags" & Chr(34) & _
":[{" & Chr(34) & "tagid" & Chr(34) & ":" & Chr(34) & "62" & Chr(34) & "," & Chr(34) & "tagstr" & Chr(34) & ":" & Chr(34) & "Example1" & Chr(34) & "},{" & Chr(34) & "tagid" & Chr(34) & ":" & Chr(34) & "3" & Chr(34) & _
"," & Chr(34) & "tagstr" & Chr(34) & ":" & Chr(34) & "Example1" & Chr(34) & "},{" & Chr(34) & "tagid" & Chr(34) & ":" & Chr(34) & "65" & Chr(34) & "," & Chr(34) & "tagstr" & Chr(34) & ":" & Chr(34) & "Example1" & Chr(34) & _
"},{" & Chr(34) & "tagid" & Chr(34) & ":" & Chr(34) & "71" & Chr(34) & "," & Chr(34) & "tagstr" & Chr(34) & ":" & Chr(34) & "Example1" & Chr(34) & "}]," & Chr(34) & "text" & Chr(34) & ":" & Chr(34) & "Example1" & Chr(34) & _
"}]," & Chr(34) & "hidden" & Chr(34) & ":" & Chr(34) & "false" & Chr(34) & "," & Chr(34) & "id" & Chr(34) & ":" & Chr(34) & "1" & Chr(34) & "," & Chr(34) & "questionalias" & Chr(34) & ":" & Chr(34) & "1" & Chr(34) & _
"," & Chr(34) & "text" & Chr(34) & ":" & Chr(34) & "Example1" & Chr(34) & "," & Chr(34) & "ttl" & Chr(34) & ":" & Chr(34) & "Example1" & Chr(34) & "}},"
Debug.Print "My data is:" & vbNewLine & Data
Set Re = New RegExp
Re.IgnoreCase = True
Re.Global = True
Re.MultiLine = True
Re.Pattern = "(?=" & Chr(34) & "tagstr" & Chr(34) & "|" & Chr(34) & _
"text" & Chr(34) & "|" & Chr(34) & "ttl" & Chr(34) & ")(?:" & Chr(34) & _
"\w*" & Chr(34) & ":" & Chr(34) & "(.*?)" & Chr(34) & ")"
Debug.Print "My pattern is:" & vbNewLine & Re.Pattern
Set ReMatch = Re.Execute(Data)
Debug.Print "Matched " & ReMatch.Count & " times!"
For Each CurrentMatch In ReMatch
Debug.Print "Capture " & CurrentMatch.SubMatches(0) & " in " & CurrentMatch.Value
Next
End Sub
Output:
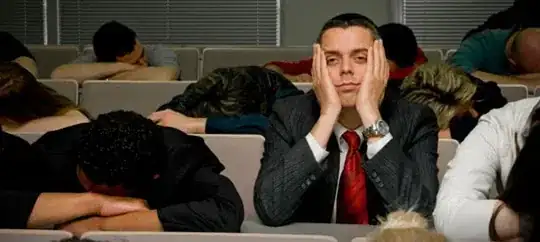
Not so complicated, right?
You can do this with standart string functions after all..