You can create a custom view. It's pretty easy. You can give the underline any thickness you want.
To create a customView:
CustomView.java
package com.rachit.customview;
import android.content.Context;
import android.content.res.TypedArray;
import android.graphics.Canvas;
import android.graphics.Color;
import android.graphics.Paint;
import android.graphics.Paint.Style;
import android.util.AttributeSet;
import android.view.View;
/**
* Custom View
* Created by Rachit on 18-Apr-16.
*/
public class CustomView extends View {
// Label text
private String viewText;
// Underline Thickness
private float underlineThickness;
// Paint for drawing custom view
private Paint viewPaint;
public CustomView(Context context, AttributeSet attributeSet) {
super(context, attributeSet);
viewPaint = new Paint();
// Get the attributes specified in attrs.xml using the name we included
TypedArray typedArray = context.getTheme().obtainStyledAttributes(attributeSet, R.styleable.CustomView, 0, 0);
try {
// Get the text and colors specified using the names in attrs.xml
viewText = typedArray.getString(R.styleable.CustomView_viewText);
underlineThickness = typedArray.getDimension(R.styleable.CustomView_underlineThickness, 1f);
} finally {
typedArray.recycle();
}
}
@Override
protected void onDraw(Canvas canvas) {
// Draw the View
// Drawing the text on the view
viewPaint.setColor(Color.BLACK); // Set Text color to whatever you want
viewPaint.setTextSize(50); // Set Text Size to whatever you want
canvas.drawText(viewText, getX() + 5, getMeasuredHeight() / 2 + 20, viewPaint); // 5 and 20 are the left and top padding, you can customize that too.
viewPaint.setColor(Color.BLACK); // Set Underline color to whatever you want
canvas.drawRect(getX(), getMeasuredHeight() - underlineThickness, getX() + getMeasuredWidth(), getMeasuredHeight(),
viewPaint); // Set the start and end points of the Underline
}
public String getViewText() {
return viewText;
}
public void setViewText(String viewText) {
this.viewText = viewText;
invalidate();
requestLayout();
}
public float getUnderlineThickness() {
return underlineThickness;
}
public void setUnderlineThickness(float underlineThickness) {
this.underlineThickness = underlineThickness;
invalidate();
requestLayout();
}
}
attrs.xml in the res/values
folder
<?xml version="1.0" encoding="utf-8"?>
<resources>
<declare-styleable name="CustomView">
<attr name="viewText" format="string" />
<attr name="underlineThickness" format="dimension" />
</declare-styleable>
</resources>
Now you can define your view in the XML like this:
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:custom="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.rachit.customview.MainActivity">
<Button
android:id="@+id/change"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Change View" />
<com.rachit.customview.CustomView
android:id="@+id/customView"
android:layout_width="wrap_content"
android:layout_height="35dp"
android:layout_marginTop="30dp"
custom:underlineThickness="5dp"
custom:viewText="My View" />
</LinearLayout>
And you can even control the View programmatically:
MainActivity.java
package com.rachit.customview;
import android.graphics.Color;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.view.View;
import android.widget.Button;
import java.util.Random;
public class MainActivity extends AppCompatActivity {
CustomView customView;
Button change;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
customView = (CustomView) findViewById(R.id.customView);
change = (Button) findViewById(R.id.change);
change.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
customView.setViewText("Wassup??");
customView.setUnderlineThickness(1.0f);
}
});
}
}
The output looks like this:
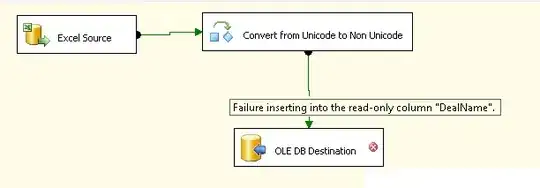
And on clicking the button, the view can be modified programmatically:
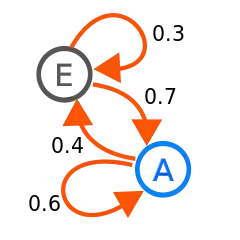