Batch Script Get Total Sum by Size and Count of Files within a Folder
Attached is a batch script method that will get you the KB, MB, and GB along with all the other detail you needed from your script. It'll all go to the log file and be appended accordingly while still iterating through the file list of servers getting the sum and counts of the files within the directory.
Batch Script
Set the LogFile
and ServerList
variables up top. Set the directory to get the file detail from within the FOR loop still as an argument passed with the call command passing that as an argument tp the dynamic PowerShell script build routine that does the calculations, etc.
@ECHO ON
SET LogFile=filecount.TXT
SET ServerList=Servers.txt
ECHO.........................................>> "%LogFile%"
ECHO DATE: %DATE% >> "%LogFile%"
ECHO TIME: %TIME% >> "%LogFile%"
ECHO USER: %USERNAME% >> "%LogFile%"
ECHO COMPUTER: %COMPUTERNAME% >> "%LogFile%"
ECHO. >> "%LogFile%"
FOR /F "TOKENS=1" %%i IN (%ServerList%) DO (
ECHO ~~~Counting %%~i~~~ >> "%LogFile%"
CALL :PowerShellBuild "\\%%~i\c$\folder1\folder2"
)
ECHO.........................................>> "%LogFile%"
EXIT
GOTO EOF
:::::::::::::::::::::::: END OF SCRIPT STILL :::::::::::::::::::::::::
:PowerShellBuild
SET TmpPSScript=%Temp%\~tmpFileCalc.ps1
IF EXIST "%TmpPSScript%" DEL /Q /F "%TmpPSScript%"
ECHO $Folder = "%~1" >>"%TmpPSScript%"
ECHO $Files = Get-ChildItem $folder>>"%TmpPSScript%"
ECHO $KBTotal = ($files ^| Measure-Object -Sum Length).Sum / 1kb >>"%TmpPSScript%"
ECHO $MBTotal = ($files ^| Measure-Object -Sum Length).Sum / 1mb >>"%TmpPSScript%"
ECHO $GBTotal = ($files ^| Measure-Object -Sum Length).Sum / 1gb >>"%TmpPSScript%"
ECHO $Count = ($files ^| Measure-Object).count >>"%TmpPSScript%"
ECHO $KBTotal = [math]::Round($KBTotal, 2) >>"%TmpPSScript%"
ECHO $MBTotal = [math]::Round($MBTotal, 2) >>"%TmpPSScript%"
ECHO $GBTotal = [math]::Round($GBTotal, 2) >>"%TmpPSScript%"
ECHO ECHO "Count: $Count" ^| Out-File -encoding ascii -append "%LogFile%" >>"%TmpPSScript%"
ECHO ECHO "KB: $KBTotal" ^| Out-File -encoding ascii -append "%LogFile%" >>"%TmpPSScript%"
ECHO ECHO "MB: $MBTotal" ^| Out-File -encoding ascii -append "%LogFile%" >>"%TmpPSScript%"
ECHO ECHO "GB: $GBTotal" ^| Out-File -encoding ascii -append "%LogFile%" >>"%TmpPSScript%"
:PowerShellExec
SET PowerShellDir=C:\Windows\System32\WindowsPowerShell\v1.0
CD /D "%PowerShellDir%"
Powershell -ExecutionPolicy Bypass -Command "& '%TmpPSScript%'"
GOTO EOF
My Test Example Script
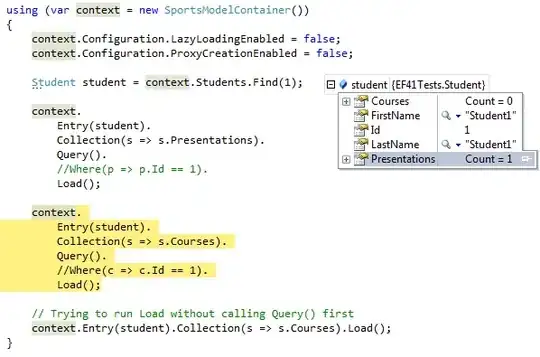
Result
........................................
DATE: Sat 03/11/2017
TIME: 16:52:32.48
USER: User
COMPUTER: FBIPC
~~~Counting Clutter~~~
Count: 15
KB: 2490.48
MB: 2.43
GB: 0
~~~Counting Photowork~~~
Count: 7
KB: 372.88
MB: 0.36
GB: 0
........................................
Native PowerShell
Test the syntax on your system(s) with the native PowerShell to confirm it works at this level from the PowerShell ISE app but it works for my with Windows 10 with PowerShell 5.0 and Windows 7 with PowerShell 4.0
$LogFile = "C:\Users\User\Desktop\Scripts\logfile.log"
$Folder = "C:\Users\User\Desktop\Scripts"
$Files = Get-ChildItem $folder
$KBTotal = ($files | Measure-Object -Sum Length).Sum / 1kb
$MBTotal = ($files | Measure-Object -Sum Length).Sum / 1mb
$GBTotal = ($files | Measure-Object -Sum Length).Sum / 1gb
$Count = ($files | Measure-Object).count
$KBTotal = [math]::Round($KBTotal, 2)
$MBTotal = [math]::Round($MBTotal, 2)
$GBTotal = [math]::Round($GBTotal, 2)
ECHO "Count: $Count" | Out-File -encoding ascii -append $Logfile
ECHO "KB: $KBTotal" | Out-File -encoding ascii -append $Logfile
ECHO "MB: $MBTotal" | Out-File -encoding ascii -append $Logfile
ECHO "GB: $GBTotal" | Out-File -encoding ascii -append $Logfile
Further Resources