That would be a very nice feature to be added in the core of Django's admin. Until then here's a quick walkthrough to your question.
Let's assume that you want to print the docstring
of each model, like this:
class MyModel(models.Model):
"""
I wanna get printed in the Admin!
"""
# model fields here
So, you want to print it in the change_list
page. Good.
Create a custom template tag (either within your app or create another app that will hold the global template tags/filters) like this:
from django import template
from django.utils.html import mark_safe
register = template.Library()
@register.simple_tag()
def model_desc(obj):
if obj.__doc__:
return mark_safe('<p>{}</p>'.format(obj.__doc__))
return ''
Now, inside your project directory (where manage.py
lives) create a structure like this:
project/
project/
project stuff here, i.e wsgi.py, settings etc
myapp/
myapp stuff here, i.e models, views etc
templates/
admin/
change_list.html
manage.py
Inside the change_list.html
add these:
{% extends 'admin/change_list.html' %}
{% load yourapp_tags %}
{# block.super will print the "Select <Model> to change" h1 title #}
{# The model_desc template tag is the one you created and prints the docstring of the given model #}
{% block content_title %}{{ block.super }}<br>{% model_desc cl.model %}{% endblock %}
Here's a screenshot:
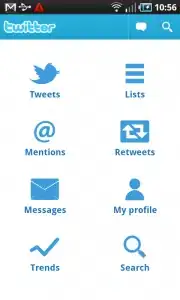
[UPDATE]: I have seen in the source that when there is no docstring
specified, Django will generate one for you in the following form: ModelName(model_field_name1, model_field_name2, ...)
. If you do not want that, simply do this:
class MyModelWithoutDocstring(models.Model):
# model fields here
MyModelWithoutDocstring.__doc__ = '' # "reset" the __doc__ on this model.