** Update Start **
I realize now you also want the url to exist so that you can allow people to go directly to the location with the URL. You will also need to handle a function that will pull the Parameters from the URL.
I believe this answer will help you with that, but I have not faced that problem yet.
** Update End **
The website you are trying to replicate the functionality of is making a get request.
In angular 2 if you want to pass data to the url you would do it like this,
You will need these imports
import { Http, Response, Headers, URLSearchParams }from '@angular/http';
import { Observable }from 'rxjs/Observable';
import 'rxjs/add/operator/do';
import 'rxjs/add/operator/take';
Create observable to update the data when it changes in the page without refreshing it.
private DimensionsData: Observable<any>;
This function gets called everytime there is a new data set to pass.
private SendData() {
let params: URLSearchParams = new URLSearchParams();
params.set('width', this.width);
params.set('length', this.length);
return this.http.get('UrlPathHere/',
{ search: params })
.map((res: Response) => res.json())
.take(1) //Takes the first observable and unsubscribes
.subscribe(res => this.DimensionsData = res.data_response)
}
You will call the function to get things started in the ngOnInit() method,
ngOnInit() {
this.SendData();
}
Now you can take DimensionsData
and use it in your code as the settings on the page. Every time someone updates the page, the GET
request would get called and this variable
would update. DimensionsData
.
All you have to do is create a service that takes the data from the URL
and then passes it back as the response
.
I have explained how to do this with a get request because that is exactly how the example website is doing it that you showed me.
You can see that in this picture here,
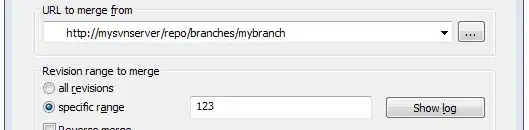