In the beginning, let's say there are 10 numbers in the list from 0 to 9.
0 1 2 3 4 5 6 7 8 9
Just like in normal weighted union-find, each of these numbers is an array index and the content of the array index value represents the parent of the array index.
So, initially, the parent of 0 is 0 and the root of 0 (the grandest of grandparents) is also 0. The same is true for all numbers.
Now, we remove a number, say 5.
Removing 5 means, we are actually saying union (5, 6).
So, this is happening.
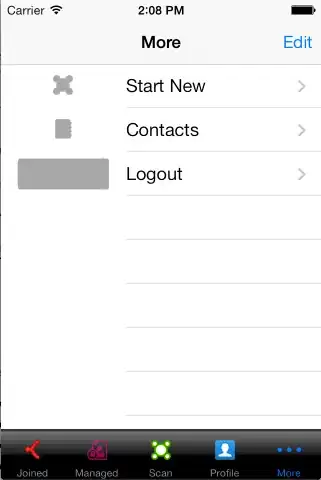
At this stage, if we want to find the successor of a number x, we can simply find it as root (x+1). So, the successor of 4 is 6, because root (4+1) is 6.
Now, let's say we remove 6.This means union (6, 7).
This is tricky because in weighted union-find, the root of 7 (7) should be added to the root of 6 (6) as the 6-5 component has more weight. But if we do that, how will we find the successor? Because this will happen:

So, if want the successor of 4, we can't say it is root (4+1) as root (5) is 6 but 6 has been removed. The successor of 4 should be 7.
So, we can use another array called, say, actualList. This array will store the actual number that needs to be on our list - that corresponds to the root of any removed number. One line of modification will be needed in union() to do this.
In this case, the actualList array will store 7 corresponding to the index root(5) and root(6). So, actualList[root(4+1)] will yield the correct answer of the successor of 4 to be 7.
To find the successor, we'll have to access actualList[(root(x+1)] and not root (x+1).
Here's my implementation of the whole thing in Java:
public class SuccessorWithDelete {
private int id[];
private int sz[];
private int actualList[];
private int N;
public SuccessorWithDelete(int N){
this.N = N;
id = new int[N];
sz = new int[N];
actualList = new int[N];
for(int i=0; i<N; i++){
id[i] = i;
sz[i] = 1;
actualList[i] = i;
}
}
// returns the root of the component the integer is in
private int root(int i){
while(id[i]!=i){
i = id[i];
}
return i;
}
// weighted quick union
public void union(Integer p, Integer q) {
int pRoot = root(p);
int qRoot = root(q);
if (sz[pRoot] < sz[qRoot]) {
id[pRoot] = qRoot;
sz[qRoot] = sz[qRoot] + sz[pRoot];
} else {
id[qRoot] = pRoot;
sz[pRoot] = sz[pRoot] + sz[qRoot];
actualList[pRoot] = qRoot; // this is the crucial step
}
}
public void remove(int x){
union(x, x+1);
}
public int successor(int x){
return actualList[(root(x+1))];
}
}