You can use Canvas
to draw a View
by the following code:
public Bitmap createViewBitmap(View v)
{
Bitmap bitmap = Bitmap.CreateBitmap(v.Width, v.Height,
Bitmap.Config.Argb8888);
Canvas canvas = new Canvas(bitmap);
v.Draw(canvas);
return bitmap;
}
Linear layout is a kind of View
. So you can create a Linear layout BitMap :
View v = FindViewById<LinearLayout>(Resource.Id.myLinearLayout);
Bitmap myBitMap = createViewBitmap(v);
And then save it in the DCIM folder:
public static void saveImage(Bitmap bmp)
{
try
{
using (var os = new System.IO.FileStream(Android.OS.Environment.ExternalStorageDirectory + "/DCIM/Camera/MikeBitMap2.jpg", System.IO.FileMode.CreateNew))
{
bmp.Compress(Bitmap.CompressFormat.Jpeg, 95, os);
}
}
catch (Exception e)
{
}
}
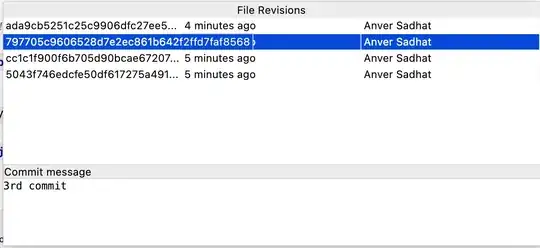
You can refer to my github for the more code information.