Use the JSON smart library that comes with JMeter. For sample code, refer the following answer or the one given by the previous answer.
You can then use vars.putObject and vars.getObject to store and retrieve objects and pass them around between samplers.
https://stackoverflow.com/a/42859821/7332423
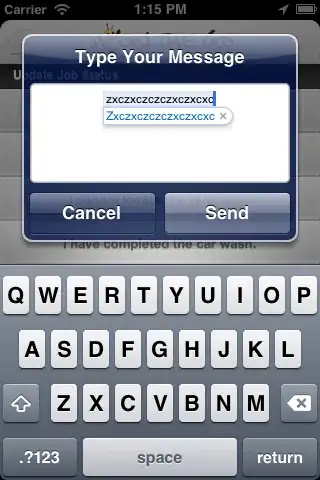
Jmeter test plan test.jmx
<?xml version="1.0" encoding="UTF-8"?>
<jmeterTestPlan version="1.2" properties="2.9" jmeter="3.0 r1743807">
<hashTree>
<TestPlan guiclass="TestPlanGui" testclass="TestPlan" testname="Test Plan" enabled="true">
<stringProp name="TestPlan.comments"></stringProp>
<boolProp name="TestPlan.functional_mode">false</boolProp>
<boolProp name="TestPlan.serialize_threadgroups">false</boolProp>
<elementProp name="TestPlan.user_defined_variables" elementType="Arguments" guiclass="ArgumentsPanel" testclass="Arguments" testname="User Defined Variables" enabled="true">
<collectionProp name="Arguments.arguments"/>
</elementProp>
<stringProp name="TestPlan.user_define_classpath"></stringProp>
</TestPlan>
<hashTree>
<ThreadGroup guiclass="ThreadGroupGui" testclass="ThreadGroup" testname="Thread Group" enabled="true">
<stringProp name="ThreadGroup.on_sample_error">continue</stringProp>
<elementProp name="ThreadGroup.main_controller" elementType="LoopController" guiclass="LoopControlPanel" testclass="LoopController" testname="Loop Controller" enabled="true">
<boolProp name="LoopController.continue_forever">false</boolProp>
<stringProp name="LoopController.loops">1</stringProp>
</elementProp>
<stringProp name="ThreadGroup.num_threads">1</stringProp>
<stringProp name="ThreadGroup.ramp_time">1</stringProp>
<longProp name="ThreadGroup.start_time">1490275382000</longProp>
<longProp name="ThreadGroup.end_time">1490275382000</longProp>
<boolProp name="ThreadGroup.scheduler">false</boolProp>
<stringProp name="ThreadGroup.duration"></stringProp>
<stringProp name="ThreadGroup.delay"></stringProp>
</ThreadGroup>
<hashTree>
<DebugSampler guiclass="TestBeanGUI" testclass="DebugSampler" testname="Debug Sampler" enabled="true">
<boolProp name="displayJMeterProperties">false</boolProp>
<boolProp name="displayJMeterVariables">true</boolProp>
<boolProp name="displaySystemProperties">false</boolProp>
</DebugSampler>
<hashTree>
<BeanShellPreProcessor guiclass="TestBeanGUI" testclass="BeanShellPreProcessor" testname="BeanShell PreProcessor" enabled="true">
<stringProp name="filename"></stringProp>
<stringProp name="parameters"></stringProp>
<boolProp name="resetInterpreter">false</boolProp>
<stringProp name="script">import org.apache.commons.lang3.StringUtils;
import net.minidev.json.parser.JSONParser;
import net.minidev.json.JSONObject;
import net.minidev.json.JSONArray;
String sInputString = "return {\"items\":[{\"STANDARDRATEFORMAT\":\"0.00\",\"ASSIGNED_HRS\":0,\"RESOURCE_NAME\":\"#Buddhika \",\"COST\":\"0.00\",\"PERCENTASSIGNED\":\"100.00\",\"EMAIL\":\"Buddhika75@mspblank.com\",\"AVAILABLEFROM\":\"10-May-2011\",\"ALLOCATED_HRS\":\"1872.00\",\"RESOURCE_ID\":36197221,\"AVAILABLETO\":\"31-Mar-2012\",\"calendar\":{\"exceptions\":{},\"weekDayHours\":{}}}]}";
//String sInputString = prev.getResponseDataAsString();
try {
// Use StringUtils to cut the string between the two
String sCutString = StringUtils.substringBetween(sInputString, "return {\"items", "]}");
String sFinalString = "{\"items" + sCutString + "]}";
log.info("sFinalString=" + sFinalString);
// Use JSONParser to parse the JSON
JSONParser parser = new JSONParser(JSONParser.ACCEPT_NON_QUOTE|JSONParser.ACCEPT_SIMPLE_QUOTE);
JSONObject rootObject = (JSONObject) parser.parse(sFinalString);
//JSONObject rootObject = (JSONObject) parser.parse(prev.getResponseDataAsString());
JSONArray jResourceArray = (JSONArray) rootObject.get("items");
for (int i=0; i < jResourceArray.size(); i++) {
log.info(jResourceArray.get(i).toString());
// You can access individual elements using this
log.info("RESOURCE_ID=" + jResourceArray.get(i).get("RESOURCE_ID"));
}
}
catch ( Exception ex) {
log.info("Exception.." + ex);
}</stringProp>
</BeanShellPreProcessor>
<hashTree/>
</hashTree>
</hashTree>
</hashTree>
</hashTree>
</jmeterTestPlan>