There's no trackbar control in Inno Setup.
But you can draw your own interactive volume bar using TBitmapImage
:
function GetCursorPos(var lpPoint: TPoint): BOOL;
external 'GetCursorPos@user32.dll stdcall';
function ScreenToClient(hWnd: HWND; var lpPoint: TPoint): BOOL;
external 'ScreenToClient@user32.dll stdcall';
procedure DrawVolume(Image: TBitmapImage; Volume: Integer);
var
Canvas: TCanvas;
Width: Integer;
begin
Canvas := Image.Bitmap.Canvas;
Canvas.Pen.Style := psClear;
Width := Image.Bitmap.Width * Volume / 100
Canvas.Brush.Color := clHighlight;
Canvas.Rectangle(1, 1, Width, Image.Bitmap.Height);
Canvas.Brush.Color := clBtnFace;
Canvas.Rectangle(Width - 1, 1, Image.Bitmap.Width, Image.Bitmap.Height);
Canvas.Pen.Style := psSolid;
Canvas.Pen.Mode := pmCopy;
Canvas.Pen.Color := clBlack;
Canvas.Brush.Style := bsClear;
Canvas.Rectangle(1, 1, Image.Bitmap.Width, Image.Bitmap.Height);
end;
procedure VolumeBarImageClick(Sender: TObject);
var
P: TPoint;
Image: TBitmapImage;
Volume: Integer;
begin
{ Calculate where in the bar did user click to }
GetCursorPos(P);
Image := TBitmapImage(Sender);
ScreenToClient(Image.Parent.Handle, P);
Volume := ((P.X - Image.Left) * 100 / Image.Width) + 1;
{ Update volume bar }
DrawVolume(Image, Volume);
{ Replace this with your code that sets the volume }
MsgBox(Format('Setting volume to %d%%', [Volume]), mbInformation, MB_OK);
end;
procedure InitializeWizard();
var
VolumeBarImage: TBitmapImage;
begin
VolumeBarImage := TBitmapImage.Create(WizardForm);
VolumeBarImage.Parent := WizardForm;
VolumeBarImage.Left := ScaleX(10);
VolumeBarImage.Top := WizardForm.ClientHeight - ScaleY(34);
VolumeBarImage.Width := ScaleX(200);
VolumeBarImage.Height := ScaleY(20);
VolumeBarImage.BackColor := clWhite;
VolumeBarImage.Bitmap.Width := VolumeBarImage.Width;
VolumeBarImage.Bitmap.Height := VolumeBarImage.Height;
VolumeBarImage.OnClick := @VolumeBarImageClick;
{ Replace with setting to actual initial volume }
DrawVolume(VolumeBarImage, 100);
end;
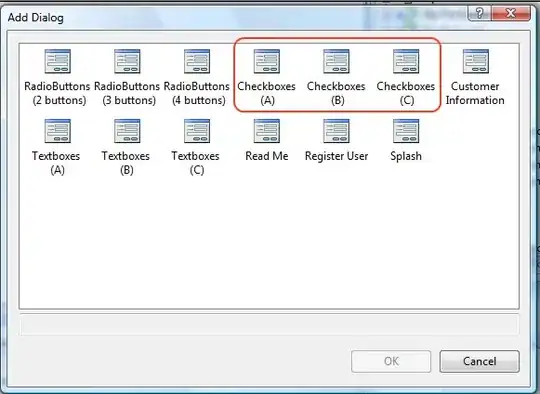
The code is mostly from: