By default imshow axes have an equal aspect ratio. To preserve this, the limits are changed.
You have two options:
a) Dispense with equal aspect
Set the aspect to "auto"
. This allows the subplots to take the available space and share their axis.
import matplotlib.pyplot as plt
import numpy as np
fig, axes = plt.subplots(2, 1,sharex=True )
axes[0].imshow(np.random.random((3, 3)), aspect="auto")
axes[1].imshow(np.random.random((6, 3)), aspect="auto")
plt.show()
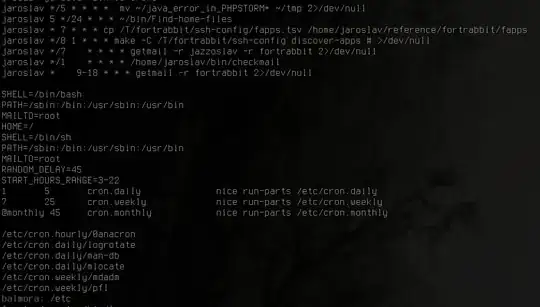
b) Adjust the figure size or spacings
You can adjust the figure size or the spacings such that the axes actually match. You'd then also need to set the height_ratios
according to the image dimensions.
import matplotlib.pyplot as plt
import numpy as np
fig, axes = plt.subplots(2, 1,sharex=True, figsize=(3,5),
gridspec_kw={"height_ratios":[1,2]} )
plt.subplots_adjust(top=0.9, bottom=0.1, left=0.295, right=0.705, hspace=0.2)
axes[0].imshow(np.random.random((3, 3)))
axes[1].imshow(np.random.random((6, 3)))
plt.show()
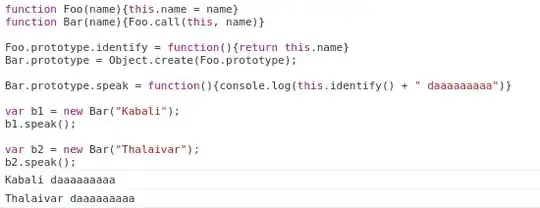
This method either involves some trial and error or a sophisticated calculation, as e.g. in this answer.