Suppose you have some code to produce a histogram like this
import matplotlib.pyplot as plt
import numpy as np; np.random.seed(0)
x = np.random.poisson(3, size=100)
p = 5.
plt.hist(x, bins=range(10))
l = plt.axvline(p, color="crimson")
legend
You can use a legend
and provide your axvline as legend handler, as well as the formatted value as legend text.
plt.legend([l], ["p={}".format(p)], loc=1)
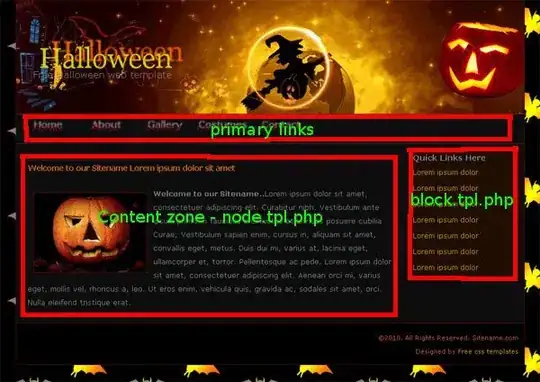
text
You can use text
to place a text in the figure. By default, the coordinates are data coordinates, but you can specify a transform to switch e.g. to axes coordinates.
plt.text(.96,.94,"p={}".format(p), bbox={'facecolor':'w','pad':5},
ha="right", va="top", transform=plt.gca().transAxes )
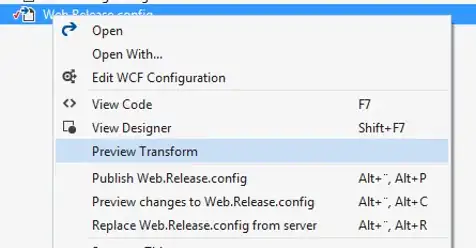
annotate
You can use annotate
to produce a text somewhere in the figure. The advantage compared to text
is that you may (a) use an additional arrow to point to an object, and (b) that you may specify the coordinate system in terms of a simple string, instead of a transform.
plt.annotate("p={}".format(p), xy=(p, 15), xytext=(.96,.94),
xycoords="data", textcoords="axes fraction",
bbox={'facecolor':'w','pad':5}, ha="right", va="top",
arrowprops=dict(facecolor='black', shrink=0.05, width=1))

AnchoredText
You can use an AnchoredText
from offsetbox:
from matplotlib.offsetbox import AnchoredText
a = AnchoredText("d={}".format(d), loc=1, pad=0.4, borderpad=0.5)
plt.gca().add_artist(a)