I needed a more general form to extract multiple paths with different features. Its a recursive solution to work with path, list of path, list of segments and single segments simultaneously. And you can specify the sample_points
per segment for curves, but a line stays 2 points to not add extra points without a need:
import svgpathtools.path
def svgpathtools_unpacker(obj, sample_points=10):
path = []
if isinstance(obj, (svgpathtools.path.Path, list)):
for i in obj:
path.extend(svgpathtools_unpacker(i, sample_points=sample_points))
elif isinstance(obj, svgpathtools.path.Line):
path.extend(obj.bpoints())
elif isinstance(obj, (svgpathtools.path.CubicBezier, svgpathtools.path.QuadraticBezier)):
path.extend(obj.points(np.linspace(0,1,sample_points)))
else:
print(type(obj))
return np.array(path)
and how you can use it:
import matplotlib.pyplot as plt
bezier_curve = svgpathtools.path.CubicBezier(start=(300+100j), control1=(100+100j), control2=(200+200j), end=(200+300j))
bezier_quad = svgpathtools.path.QuadraticBezier(bezier_curve.end, control=(200+200j), end=(300+150j))
line = svgpathtools.path.Line(start=bezier_quad.end, end=bezier_curve.start)
bezier_path = svgpathtools.path.Path(bezier_curve, bezier_quad, line)
plt.figure()
for i in [10, 100]:
xy = svgpathtools_unpacker(bezier_path, sample_points=i)
plt.plot(xy.real, xy.imag, label=f'sample_points={i}')
plt.legend()
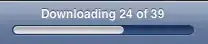