Okay, let's start with the really obvious issue, applets are effectively deprecated and you should stop using them, see Java Plugin support deprecated and Moving to a Plugin-Free Web for more details.
paintXxx
is for painting, you should never change the state of a component from within a paint method (and you should not be calling setSize
in an applet anyway). See Painting in AWT and Swing and Performing Custom Painting for more details about how painting works and how you can make use of it
As a general piece of advice, I'd recommend ImageIO.read
over Toolkit#getImage
or ImageIcon
for loading images, apart from throwing an Exception
when the image can't be read (instead of failing silently), it's also a blocking call, meaning that when it returns, the image is fully loaded.
See Reading/Loading an Image for more details
A word of warning - What you're trying to do is not difficult per se, but it's involved and requires a certain amount of knowledge about how the API works.
Now what I was planning to make is a chrome logo in the center of my screen, transparent with no background, and then chrome opens.
Okay, well, this was never going to work with applets, as applets are intended to be displayed inside a browser, so you can't control the window, instead, you need something like a JFrame
, see How to Make Frames (Main Windows)
What I want to do, however, is the make the background + the window to vanish, Once that's was done I will set the image and window size to 1920x1080. How do I go forward on making the window behind the image disappear?
Now, this is where the real fun begins. You're going to want to start by having a look at How to Create Translucent and Shaped Windows. This will allow you control the opacity of the frame, making it disappear, but allowing the content to remain, there are some tricks you need to do to pull it off, but that's the basic idea
So, this example will make a transparent window and display and image centered within it.
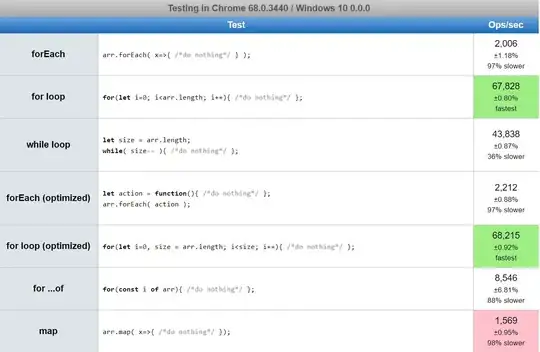
import java.awt.Color;
import java.awt.EventQueue;
import java.awt.image.BufferedImage;
import java.io.IOException;
import javax.imageio.ImageIO;
import javax.swing.ImageIcon;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.UIManager;
import javax.swing.UnsupportedLookAndFeelException;
public class Test {
public static void main(String[] args) {
new Test();
}
public Test() {
EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
try {
try {
UIManager.setLookAndFeel(UIManager.getSystemLookAndFeelClassName());
} catch (ClassNotFoundException | InstantiationException | IllegalAccessException | UnsupportedLookAndFeelException ex) {
ex.printStackTrace();
}
BufferedImage img = ImageIO.read(getClass().getResource("/Chrome.png"));
ImageIcon icon= new ImageIcon(img);
JLabel label = new JLabel(icon);
JFrame frame = new JFrame("Testing");
frame.setUndecorated(true);
frame.setBackground(new Color(0, 0, 0, 0));
frame.add(label);
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
} catch (IOException ex) {
ex.printStackTrace();
}
}
});
}
}
In this is example, I make use of a JLabel
to display the image, in most cases, it's all you really need, see How to Use Labels for more details.
What I want to do, however, is the make the background + the window to vanish
Well, it just comes down to what you mean by vanish. You could just all dispose
or setVisible
on the window, which would close it, all you could use some animation to make it fade away ... and because I like animation...
import java.awt.Color;
import java.awt.EventQueue;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.image.BufferedImage;
import java.io.IOException;
import javax.imageio.ImageIO;
import javax.swing.ImageIcon;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.Timer;
import javax.swing.UIManager;
import javax.swing.UnsupportedLookAndFeelException;
public class Test {
public static void main(String[] args) {
new Test();
}
public Test() {
EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
try {
try {
UIManager.setLookAndFeel(UIManager.getSystemLookAndFeelClassName());
} catch (ClassNotFoundException | InstantiationException | IllegalAccessException | UnsupportedLookAndFeelException ex) {
ex.printStackTrace();
}
BufferedImage img = ImageIO.read(getClass().getResource("/Chrome.png"));
ImageIcon icon = new ImageIcon(img);
JLabel label = new JLabel(icon);
JFrame frame = new JFrame("Testing");
frame.setUndecorated(true);
frame.setBackground(new Color(0, 0, 0, 0));
frame.add(label);
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
Timer timer = new Timer(40, new ActionListener() {
float opacity = 1.0f;
float delta = 0.05f;
@Override
public void actionPerformed(ActionEvent e) {
opacity -= delta;
if (opacity < 0.0f) {
opacity = 0.0f;
((Timer)(e.getSource())).stop();
frame.dispose();
}
frame.setOpacity(opacity);
}
});
timer.setInitialDelay(2000);
timer.start();
} catch (IOException ex) {
ex.printStackTrace();
}
}
});
}
}
Okay, there is a lot going on here, you'll want to have a read through Concurrency in Swing and How to use Swing Timers for more details, needless to say, it's a little complicated to get started with.
Basically, all this does is, waits 2 seconds and then every 40 milliseconds, decreases the frames opacity by a factor or 0.05
till it reaches 0
and it then disposes of the window.
Now, I might use a mixture of these techniques, for example, I might show the logo frame (maybe set to display on top), I would then show the main frame and then trigger the logo frame to fade out. This could be done from a controller class to simplify the code, but, that's up to you.
A would also suggest having a look at Creating a GUI With JFC/Swing