As I understand it, NumPy is following the axis numbering philosophy when it spits out the result when given a list/tuple
-like index.
array([[[1, 1, 1],
[2, 2, 2]],
[[3, 3, 3],
[4, 4, 4]]])
When you already specify the first two indices (x[0, :, ]
), now the next question is how to extract the third dimension. Now, when you specify a tuple (0,1)
, it first extracts the 0
th slice axis wise, so it gets [1, 2]
since it lies in 0
th axis, next it extracts 1
st slice likewise and stacks below the already existing row [1, 2]
.
[[1, 1, 1], array([[1, 2],
[2, 2, 2]] =====> [1, 2]])
(visualize this stacking as below (not on top of) the already existing row since axis-0 grows downwards)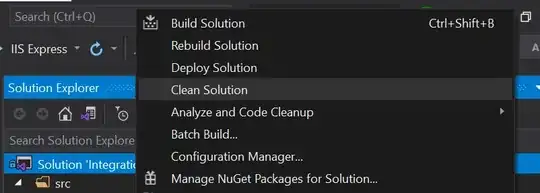
Alternatively, it is following the slicing philosophy (start
:stop
:step
) when slice(n)
is given for the index. Note that using slice(2)
is essentially equal to 0:2
in your example. So, it extracts [1, 1]
first, then [2, 2]
. Note, here to how [1, 1]
comes on top of [2, 2]
, again following the same axis philosophy here since we didn't leave the third dimension yet. This is why this result is the transpose of the other.
array([[1, 1],
[2, 2]])
Also, note that starting from 3-D arrays this consistency is preserved. Below is an example from 4-D
array and the slicing results.
In [327]: xa
Out[327]:
array([[[[ 0, 1, 2],
[ 3, 4, 5],
[ 6, 7, 8]],
[[ 9, 10, 11],
[12, 13, 14],
[15, 16, 17]]],
[[[18, 19, 20],
[21, 22, 23],
[24, 25, 26]],
[[27, 28, 29],
[30, 31, 32],
[33, 34, 35]]]])
In [328]: xa[0, 0, :, [0, 1]]
Out[328]:
array([[0, 3, 6],
[1, 4, 7]])
In [329]: xa[0, 0, :, 0:2]
Out[329]:
array([[0, 1],
[3, 4],
[6, 7]])