Try like this But code is in Swift
First Create Tableviewcontroller from Storyboard
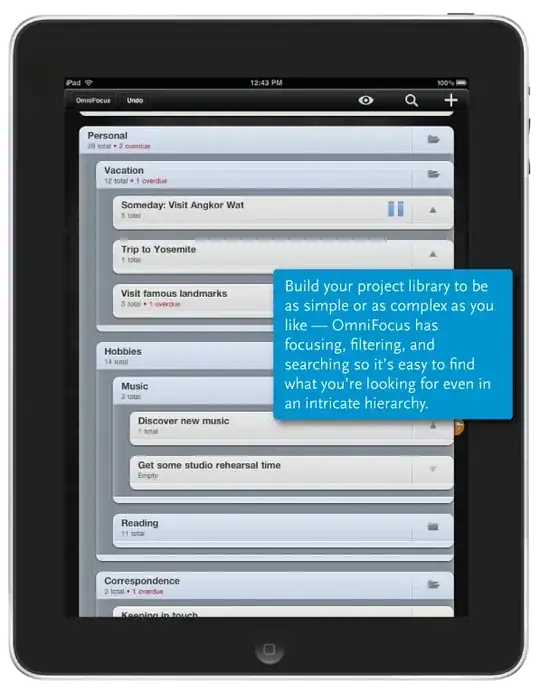
Create CustomCell of TableView
import UIKit
class CustomCell: UITableViewCell {
@IBOutlet weak var scrollView: UIScrollView!
@IBOutlet weak var pageControll: UIPageControl!
override func awakeFromNib() {
super.awakeFromNib()
}
override func setSelected(_ selected: Bool, animated: Bool) {
super.setSelected(selected, animated: animated)
}
}
Now In Tableviewcontroller and add delegate UIScrollViewDelegate,UITableViewDelegate,UITableViewDataSource
func numberOfSectionsInTableView(tableView: UITableView) -> Int {
return 1
}
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return data.count;
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell:CustomCell = tableView.dequeueReusableCell(withIdentifier: "Cell") as! CustomCell;
let scrollViewWidth:CGFloat = tableView.frame.width
let scrollViewHeight:CGFloat = cell.contentView.frame.height
let imgOne = UIImageView(frame: CGRect(x:0, y:0,width:scrollViewWidth, height:scrollViewHeight))
imgOne.image = UIImage(named: "image1")
imgOne.tag = 1
let imgTwo = UIImageView(frame: CGRect(x:scrollViewWidth, y:0,width:scrollViewWidth, height:scrollViewHeight))
imgTwo.image = UIImage(named: "image2")
imgTwo.tag = 1
cell.scrollView.addSubview(imgOne)
cell.scrollView.addSubview(imgTwo)
cell.scrollView.contentSize = CGSize(width:scrollViewWidth * 2, height:cell.scrollView.frame.height)
cell.scrollView.delegate = self
cell.pageControll.currentPage = 0
pageCtrl = cell.pageControll
return cell;
}
func scrollViewDidEndDecelerating(_ scrollView: UIScrollView){
if (scrollView.superview?.superview as? CustomCell) != nil{
let cell = scrollView.superview?.superview as! CustomCell
let pageWidth:CGFloat = self.view.frame.width
let currentPage:CGFloat = floor((scrollView.contentOffset.x - pageWidth/2)/pageWidth)+1
cell.pageControll.currentPage = Int(currentPage)
}
}