STEP 1 :
Create assets folder and in this folder create another folder fonts see below image. After creating these folders paste your ttf files in fonts folder.
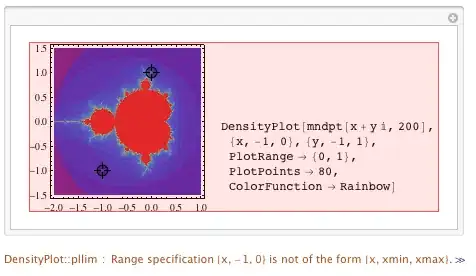
STEP 2 : Create attrs.xml file in values folder see below image and paste this code.
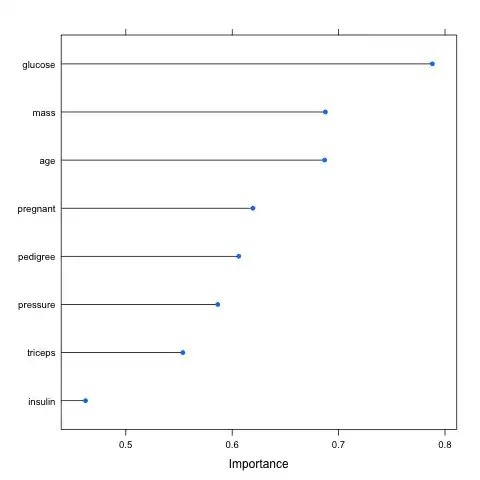
CODE
<?xml version="1.0" encoding="utf-8"?>
<resources>
<declare-styleable name="CustomTextView">
<attr name="font" format="string" />
</declare-styleable>
</resources>
STEP 3 :
Create CustomTextview.class and paste this code
import android.annotation.TargetApi;
import android.content.Context;
import android.content.res.TypedArray;
import android.graphics.Typeface;
import android.os.Build;
import android.util.AttributeSet;
import android.widget.TextView;
public class CustomTextView extends TextView {
@TargetApi(Build.VERSION_CODES.LOLLIPOP)
public CustomTextView(Context context, AttributeSet attrs, int defStyleAttr, int defStyleRes) {
super(context, attrs, defStyleAttr, defStyleRes);
init(attrs);
}
public CustomTextView(Context context, AttributeSet attrs, int defStyle) {
super(context, attrs, defStyle);
init(attrs);
}
public CustomTextView(Context context, AttributeSet attrs) {
super(context, attrs);
init(attrs);
}
public CustomTextView(Context context) {
super(context);
init(null);
}
private void init(AttributeSet attrs) {
if (attrs != null) {
TypedArray a = getContext().obtainStyledAttributes(attrs, R.styleable.CustomTextView);
String fontName = a.getString(R.styleable.CustomTextView_font);
try {
if (fontName != null) {
Typeface myTypeface = Typeface.createFromAsset(getContext().getAssets(), "fonts/" + fontName);
setTypeface(myTypeface);
}
} catch (Exception e) {
e.printStackTrace();
}
a.recycle();
}
}
}
STEP 4 :
Declare your customTextview in xml file like below.
Use this attribute app:font="your ttf file"
Note -> com.javacodegeeks.androidcustomfontexample.CustomTextView
indicate that your package name and class name.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:orientation="vertical"
android:padding="20dp">
<com.javacodegeeks.androidcustomfontexample.CustomTextView
android:id="@+id/custom_text_view"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:text="This is a custom text view!"
android:textSize="30dp"
app:font="arial.ttf" />
</LinearLayout>
YOU CAN GRAB DEMO FROM HERE
I hope it help