it is not Swift (language) specific, it is Apple specific ...

even worst if you change data to
let d = ["first":"john", "last":"doe", "test int": 0, "test null": NSNull()] as [String:Any]
linux version works as expected,
["test null": <NSNull: 0x0000000000825560>, "last": "doe", "first": "john", "test int": 0]
["test null": <NSNull: 0x0000000000825560>, "last": "doe", "first": "john", "test int": 0]
["test int": 0, "last": "doe", "first": "john", "test null": <NSNull: 0x0000000000825560>]
but apple prints
[:]
[:]
{
first = john;
last = doe;
"test int" = 0;
"test null" = "<null>";
}
it looks very strange. next code snippet explain why
import Foundation
public protocol P {}
extension String:P {}
extension Int:P {}
extension NSNull:P {}
let d = ["first":"john", "last":"doe", "test null": NSNull(), "test int": 10] as [String:Any]
print("A)",d, type(of: d))
let d1 = d as? [String:P] ?? [:]
print("B)",d1, type(of: d1))
print()
if let data = try? JSONSerialization.data(withJSONObject: d, options: []) {
if let jobject = try? JSONSerialization.jsonObject(with: data, options: []) {
let o = jobject as? [String:Any] ?? [:]
print("1)",o, type(of: o))
var o2 = o as? [String:P] ?? [:]
print("2)",o2, type(of: o2), "is it empty?: \(o2.isEmpty)")
print()
if o2.isEmpty {
o.forEach({ (t) in
let v = t.value as? P
print("-",t.value, type(of: t.value),"as? P", v as Any)
o2[t.key] = t.value as? P ?? 0
})
}
print()
print("3)",o2)
}
}
on apple it prints
A) ["test null": <null>, "test int": 10, "first": "john", "last": "doe"] Dictionary<String, Any>
B) ["test null": <null>, "test int": 10, "first": "john", "last": "doe"] Dictionary<String, P>
1) ["test null": <null>, "test int": 10, "first": john, "last": doe] Dictionary<String, Any>
2) [:] Dictionary<String, P> is it empty?: true
- <null> NSNull as? P Optional(<null>)
- 10 __NSCFNumber as? P nil
- john NSTaggedPointerString as? P nil
- doe NSTaggedPointerString as? P nil
3) ["test null": <null>, "test int": 0, "first": 0, "last": 0]
while on linux it prints
A) ["test int": 10, "last": "doe", "first": "john", "test null": <NSNull: 0x00000000019d8c40>] Dictionary<String, Any>
B) ["test int": 10, "last": "doe", "first": "john", "test null": <NSNull: 0x00000000019d8c40>] Dictionary<String, P>
1) ["test int": 10, "last": "doe", "first": "john", "test null": <NSNull: 0x00000000019ec550>] Dictionary<String, Any>
2) ["test int": 10, "last": "doe", "first": "john", "test null": <NSNull: 0x00000000019ec550>] Dictionary<String, P> is it empty?: false
3) ["test int": 10, "last": "doe", "first": "john", "test null": <NSNull: 0x00000000019ec550>]
finally, I used the slightly modified source code of JSONSerialization from open source distribution (to avoid conflict with apple Foundation I rename the class to _JSONSerialization :-) and change the code such a way it works in my Playground without any warnings and errors and ...
it prints the expected results :)
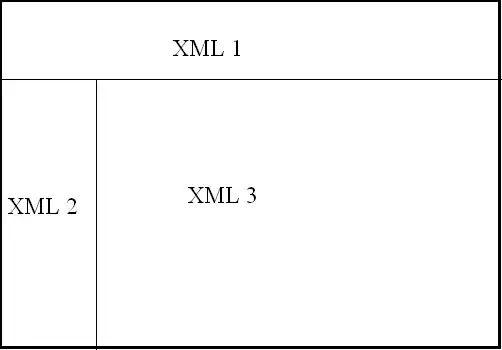
Why it works now? The key is
/* A class for converting JSON to Foundation/Swift objects and converting Foundation/Swift objects to JSON. An object that may be converted to JSON must have the following properties:
- Top level object is a `Swift.Array` or `Swift.Dictionary`
- All objects are `Swift.String`, `Foundation.NSNumber`, `Swift.Array`, `Swift.Dictionary`, or `Foundation.NSNull`
- All dictionary keys are `Swift.String`s
- `NSNumber`s are not NaN or infinity */
Now the conditional downcasting of all possible values to P works as expected
to be honest, try this snippet on linux :-) and on apple.
let d3 = [1.0, 1.0E+20]
if let data = try? JSONSerialization.data(withJSONObject: d3, options: []) {
if let jobject = try? JSONSerialization.jsonObject(with: data, options: []) as? [Double] ?? [] {
print(jobject)
}
}
apple prints
[1.0, 1e+20]
while linux
[]
and with really big value will crash. this bug goes from (in the open sourced JSONSerialization)
if doubleResult == doubleResult.rounded() {
return (Int(doubleResult), doubleDistance)
}
replace it with
if doubleResult == doubleResult.rounded() {
if doubleResult < Double(Int.max) && doubleResult > Double(Int.min) {
return (Int(doubleResult), doubleDistance)
}
}
and 'deserialization' works as expected (serialization has other bugs ...)