There is a Microsoft.Azure.Management.ServiceBus Libary that is a preview version, is 100% compatible .Netcore. We can get more detail here.
Preparetion:
Registry Azure Active Directory application and assign Role
Steps:
Create a .net core console project and add the following code.
var tenantId = "tenantid";
var context = new AuthenticationContext($"https://login.windows.net/{tenantId}");
var clientId = "Client";
var clientSecret = "Secret";
var subscriptionId = "subscriptionId";
var result = context.AcquireTokenAsync(
"https://management.core.windows.net/",
new ClientCredential(clientId, clientSecret)).Result;
var creds = new TokenCredentials(result.AccessToken);
var sbClient = new ServiceBusManagementClient(creds)
{
SubscriptionId = subscriptionId
};
var queueParams = new QueueCreateOrUpdateParameters()
{
Location = "East Asia",
EnablePartitioning = true
};
var queue = sbClient.Queues.ListAll("groupname", "namespace").ToList().FirstOrDefault(x => x.Name.Equals("queuename"));
var messagecount = queue.MessageCount;
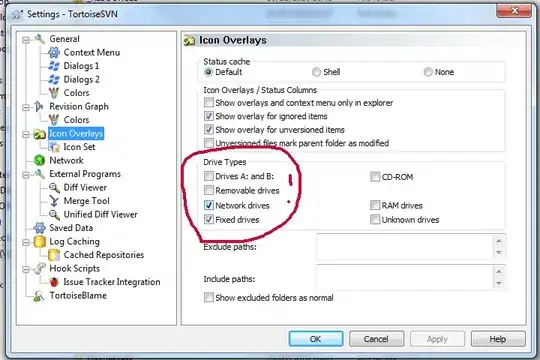
From Azure poratal, we check the message in the queue

Project.json file:
{
"version": "1.0.0-*",
"buildOptions": {
"emitEntryPoint": true
},
"dependencies": {
"Microsoft.Azure.Management.ServiceBus": "0.2.0-preview",
"Microsoft.IdentityModel.Clients.ActiveDirectory": "3.13.9",
"Microsoft.NETCore.App": {
"type": "platform",
"version": "1.0.1"
}
},
"frameworks": {
"netcoreapp1.0": {
"imports": "dnxcore50"
}
}
}